Chapter 2:
Edit Data in an Alert
We’ll next allow the user to edit the movie data. For this, we’ll use a feature of data bindings called writeback. You can toggle data binding writeback for user-editable components. This means that when a user changes a property that has a data binding, the original variable will be updated with the new value.
Step 1: Create your edit Form
Create a second Form in your app by clicking the three dots to the right of Client Code and choose Add Form. Select Blank Panel and rename it to MovieEdit
.
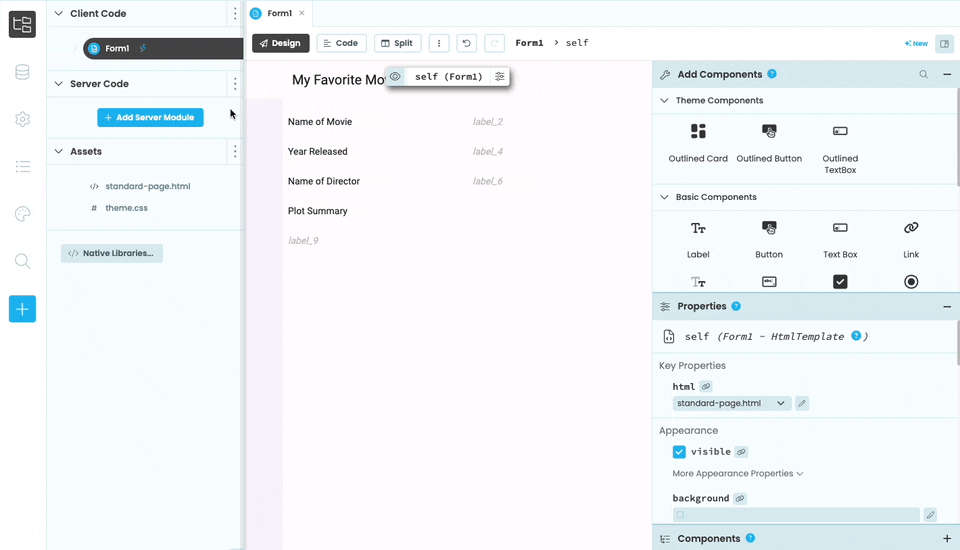
Start by dropping a ColumnPanel into the page. Setting up the Form is very similar to what we did with our previous Form, but we’ll use TextBoxes instead of Labels, since we want the user to be able to edit the fields. Make sure to change the type
propery to number
for the year of release TextBox. Use a TextArea for the plot summary since that will usually be longer than a single line. This is how it should look:
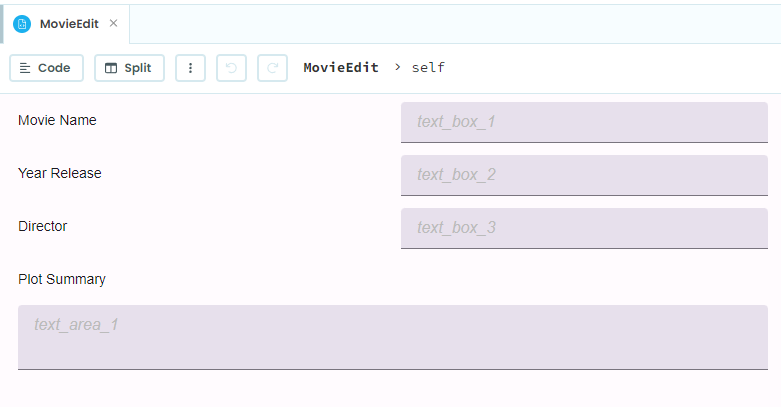
Step 2: Set up data bindings
Setting up data bindings is much the same as before. Here’s the one for text_box_1
, binding its text
property to self.item['movie_name']
:
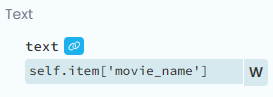
In this case, though, notice that there’s a W
at the right end of the data binding. That W
stands for writeback and will show on any property that’s user-editable. Writeback defaults to inactive, but if you click the W you can activate it.
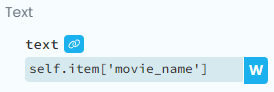
When writeback is active and the user edits the value in the Text Box, Anvil will update the original data binding source with those changes. It’s the equivalent of you executing self.item['movie_name'] = self.text_box_1.text
when the TextBox value changes.
Since our purpose is to allow the user to edit the movie information, we want to enable writeback for all of these editable components. Set up the rest, and then check them in the Form’s Data Binding summary. They should look like this:
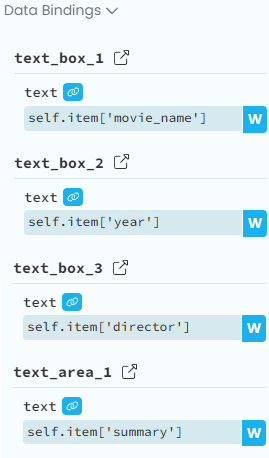
Note that we didn’t write any code in the MovieEdit Form. That’s the value of data bindings, to automate the boilerplate code of assigning values to visual components and then getting the values out when the user makes changes.
Step 3: Trigger your edit Form
We want to access our MovieEdit
Form from our main Form. We’ll do this by displaying the MovieEdit
form in an alert every time a user clicks a button.
Add a Button on Form1
. Set its text to ‘Edit movie information’ and change its name to edit_button
.
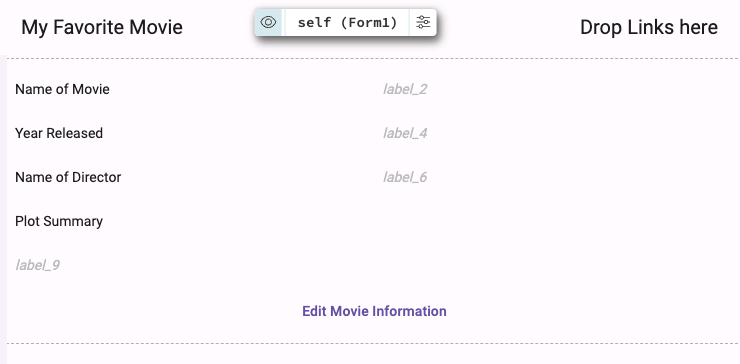
We want some code to run when this Button is clicked. We’ll use events for this. Select the Button and click on ‘on click
event’ in the Object Palette. This will open the Editor’s ‘Split’ view and automatically create an edit_button_click
method associated with the button.
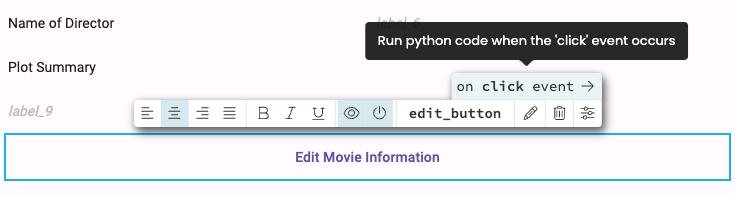
We’ll write the code that will run when we click edit_button
inside this method. First, import the MovieEdit
Form at the top of the Form:
from ..MovieEdit import MovieEdit
And then in the edit_button_click
function itself create an instance of the MovieEdit
Form:
def edit_button_click(self, **event_args):
editing_form = MovieEdit(item=self.item)
When we create the instance of the MovieEdit Form, we’re going to pass our dictionary of movie information as its item
property. That will make it available in MovieEdit
as self.item
.
Now we’re going to display the Form in an alert. We’ll set the content of the alert to be editing_form
and the large
property to be True
:
def edit_button_click(self, **event_args):
editing_form = MovieEdit(item=self.item)
alert(content=editing_form, large=True)
Run the app and click the editing button to see the movie information in the text boxes and text area. If any of the information doesn’t show up in a component, double check the data bindings.
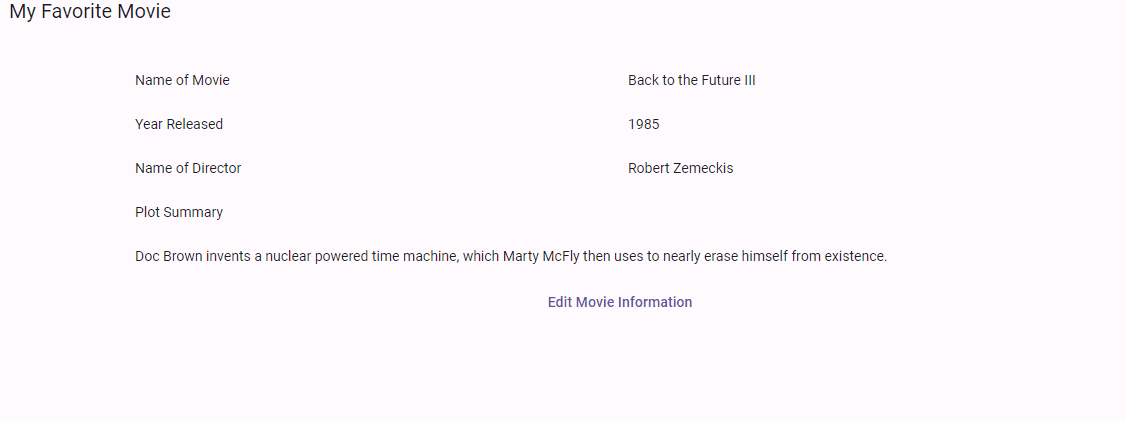
Step 4: Read the changed values
The next step is to get the changed values back into the main Form. We are actually almost there since MovieEdit
’s writeback data bindings are already changing Form1’s self.item
dictionary. But Form1 doesn’t know that those values have changed, and so doesn’t know to rerun the data bindings to update the components.
We need to tell Form1
to update its data bindings after the alert returns. We’ll do this with self.refresh_data_bindings()
:
def edit_click(self, **event_args):
editing_form = MovieEdit(item=self.item)
alert(content=editing_form, large=True)
self.refresh_data_bindings()
Run the app now and edit the movie information to see it changed in Form1
!
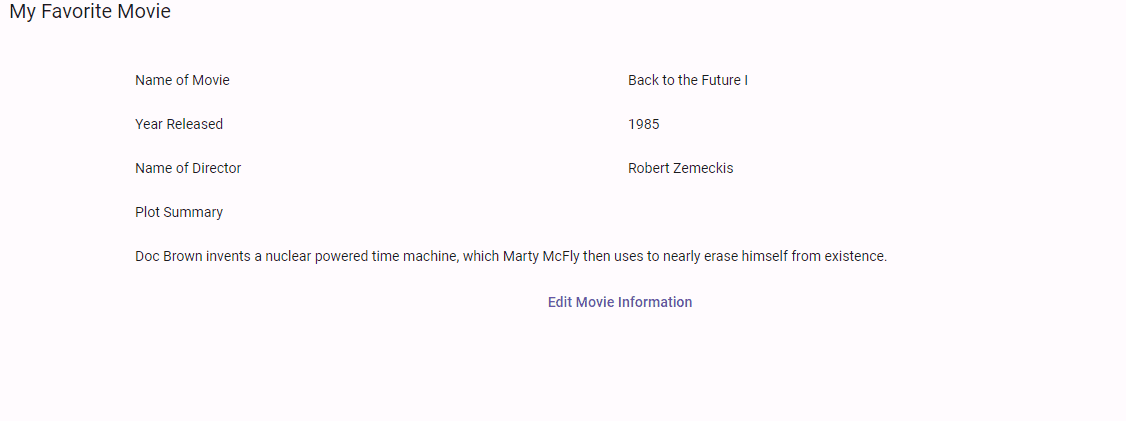
We can now edit our app’s movie data!
In the next chapter, we’ll see how to store our data into a Data Table.