Chapter 1:
Build your user interface
Let’s start by building an application form using Anvil’s drag-and-drop editor.
Note: This guide includes screenshots of the Classic Editor. Since we created this guide, we've released the new Anvil Editor, which is more powerful and easier to use.
All the code in this guide will work, but the Anvil Editor will look a little different to the screenshots you see here!
Step 1: Create your Anvil app
Creating web apps with Anvil is simple. No need to wrestle with HTML, CSS, JavaScript or PHP. We can do everything in Python.
Log in to Anvil and click ‘New Blank App’. Choose the Material Design theme.
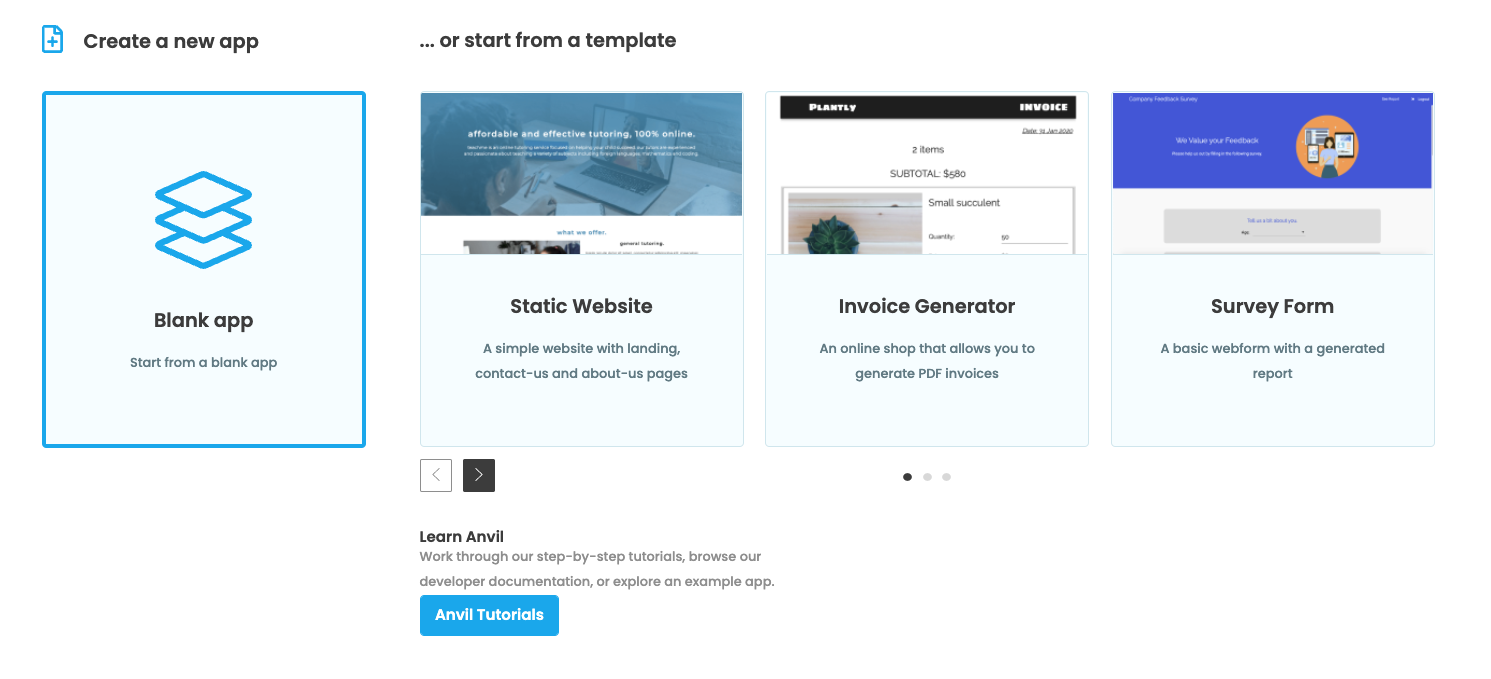
First, name the app. Click on the name at the top of the screen and give it a name.
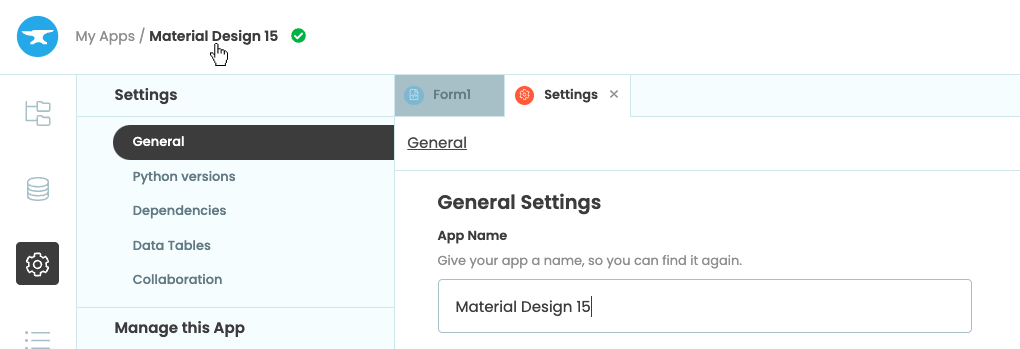
Step 2: Creating an application form and adding job details
We need to create a form for people to apply for our job.
We construct the form by dragging-and-dropping components from the Toolbox. Let’s start by dropping a Card into our form – card components provide neat containers for other components. Then let’s add a Label
to act as the title of our form. Select the Label and, in the properties panel on the right, change the name of the component to
title
, then text to the name of our company and finally change the role to Headline
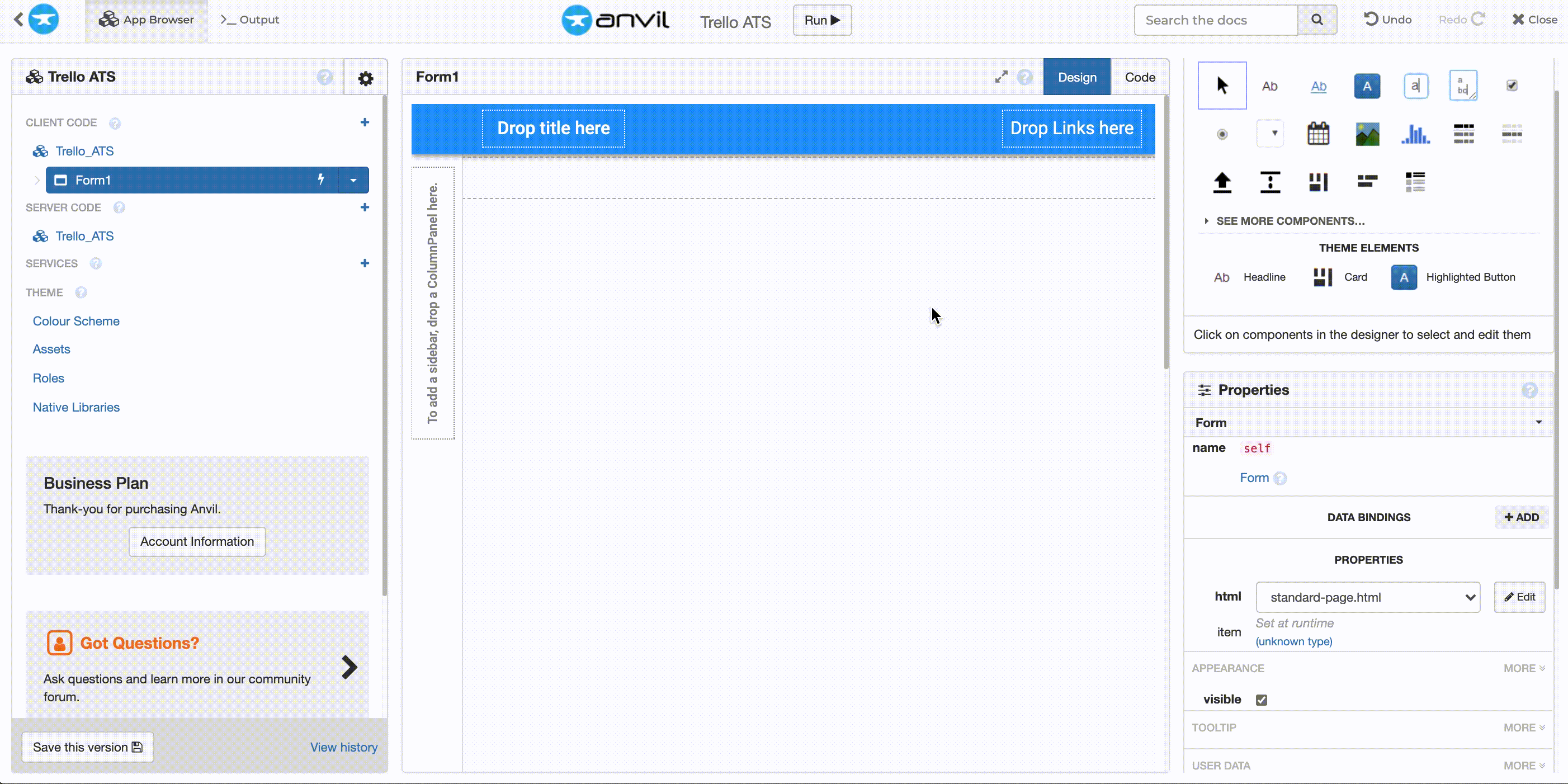
Adding a title
Now our form has a title, let’s add another Card into our form. This card will contain all of our job details and should end up looking like this:
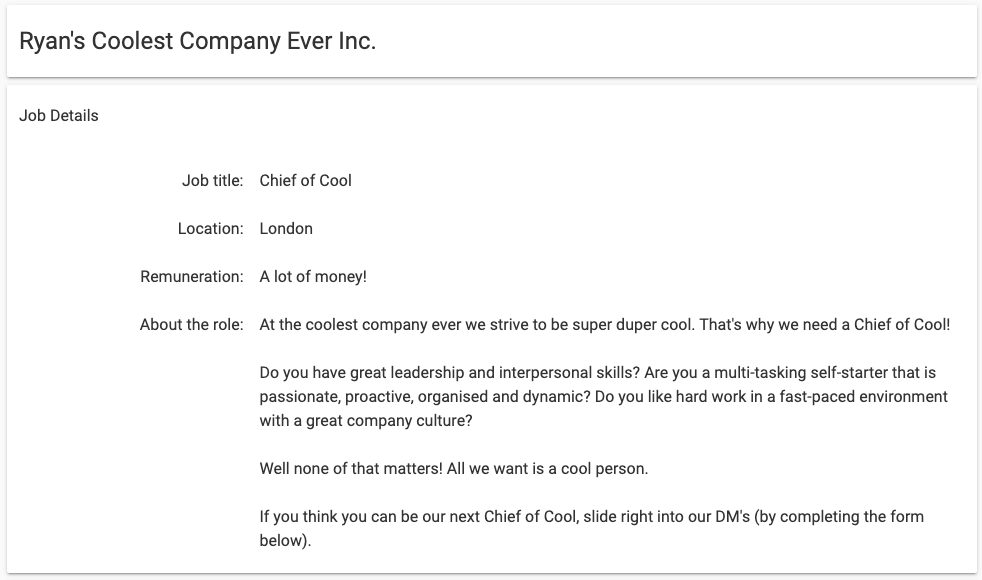
Finished job details section
Start by adding a label with the text Job details
and changing its role to subheading
.
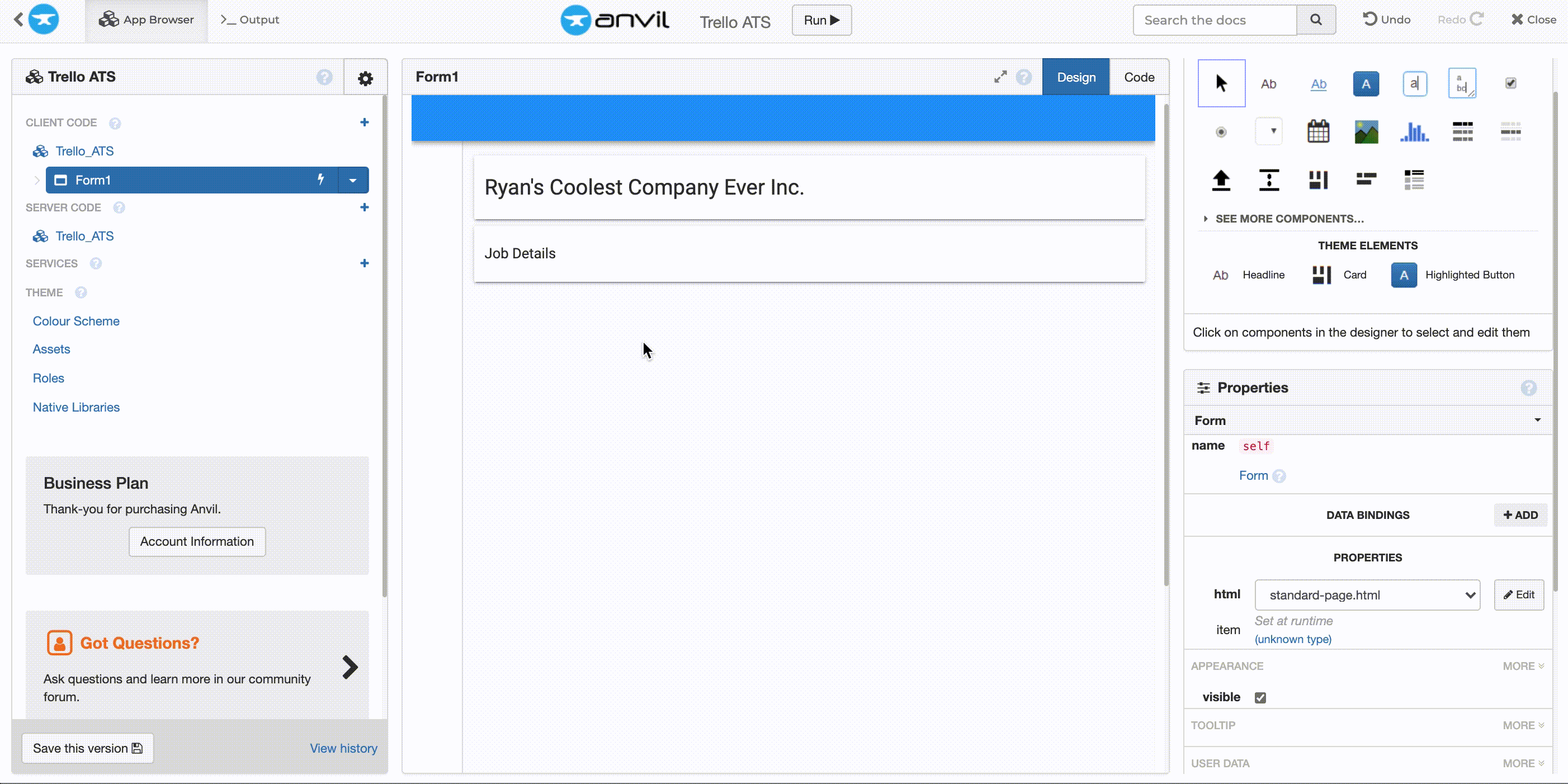
Adding a subheading
Then, add a Label with the text
Job title:
, align it to the right and change it’s role to input-prompt
. Drag and drop another label to the right of the Job title:
Label and change it’s text to the title of your job vacancy. Repeat this process adding information for:
Location
Remuneration
About the role
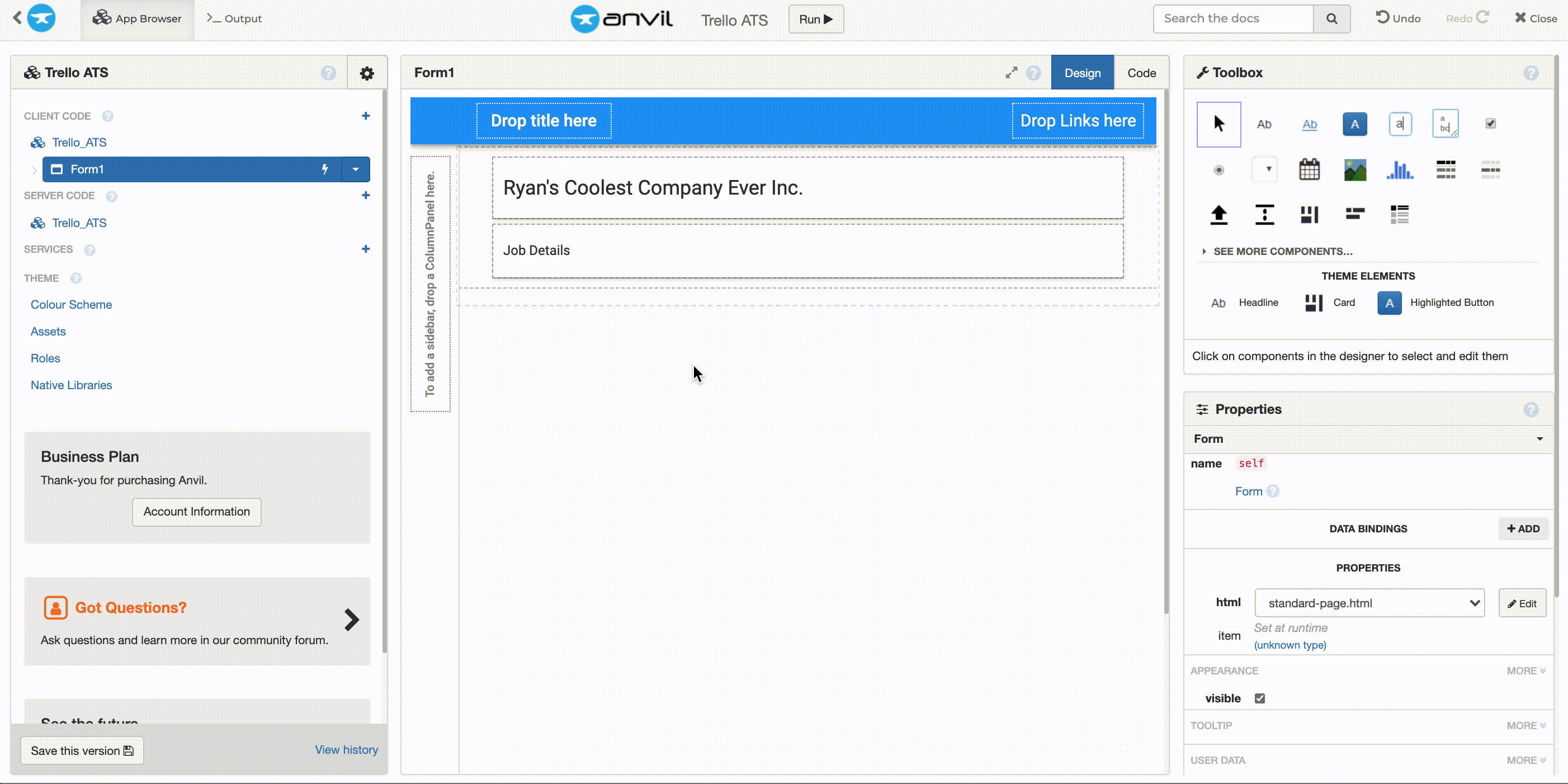
Adding job details
Now we have our job details, let’s add input fields for people to complete their application.
Step 3: Adding input fields to our application form
We need people to be able to enter their details for the job application, so let’s add input fields to our form.
Our finished form will look like this:
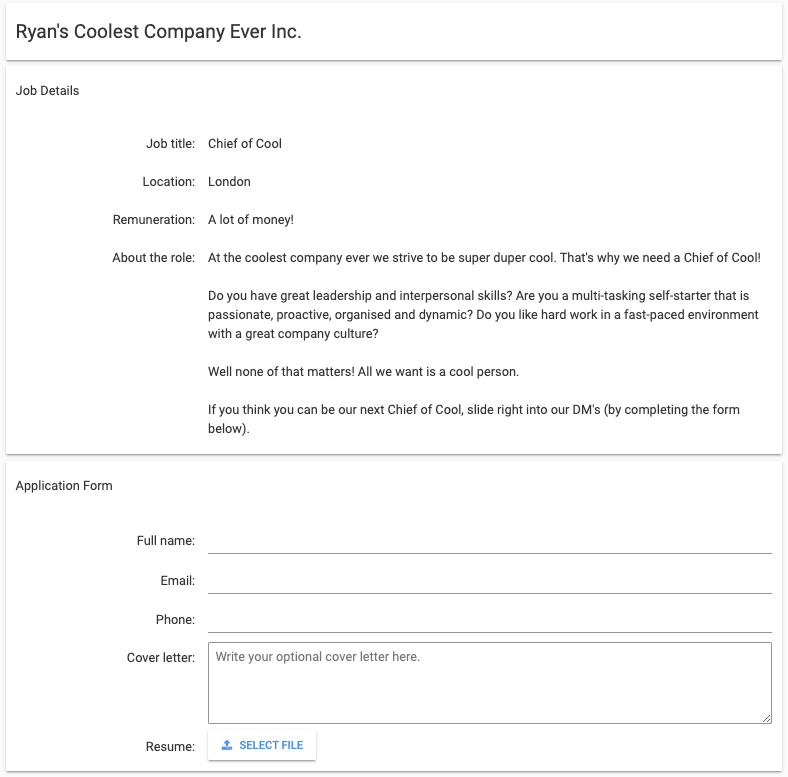
Finished input fields
Add another Card and then a Label with the text Application Form
and role subheading
.
Then add a Label and add a TextBox component to the right of the label. Select the Label and change the Label’s text to
Full name:
, align it to the right and set its role to input-prompt
. Select the TextBox and change its name to name_text_box
. Repeat this process adding the following fields:
- An
Email:
Label next to a TextBox namedemail_text_box
, set the TextBox’s type toemail
. - A
Phone:
Label next to a TextBox namedphone_text_box
, set the TextBox’s type tonumber
. - A
Cover letter:
Label next to a TextArea namedcover_letter_text_area
, set the TextArea’s placeholder text to ‘Write your optional cover letter here’.
Lastly, add a Label with the text Resume:
. Next to the Label, add a FileLoader component . Change its name to
resume_file_uploader
, its text to Select file
and its role to raised
.
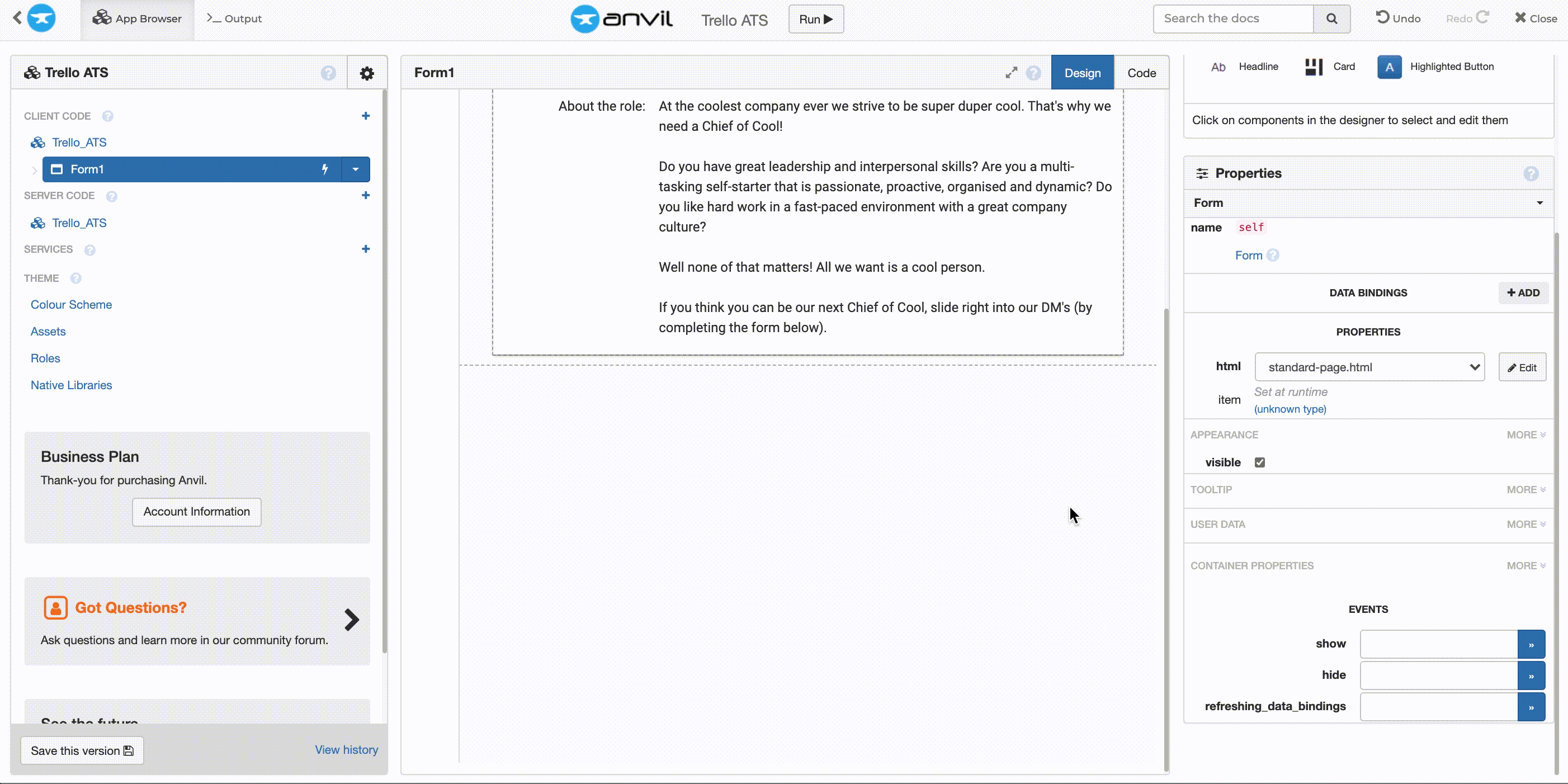
Adding input fields
Now our form can collect all the data we need for our applicants, let’s add a submit button which will send this data to our Trello Board.
That’s it, we’ve created a form for our users to apply for a job at our company.
In Chapter 2, we’ll create a Trello board which we’ll use to organise and track our job applications.