Chapter 2:
Make the User Interface
Now it’s time to add a proper display and button to our app. When clicked, the button will display a random book from the library collection in our Sheet.
We’ll make it work by writing some more client-side Python code that runs in the browser.
Step 1: Add a title
First, let’s give the app a title. We’ll call it BookFinder! Drag a new Label on to the “Drop Title Here” area in the Form Editor.
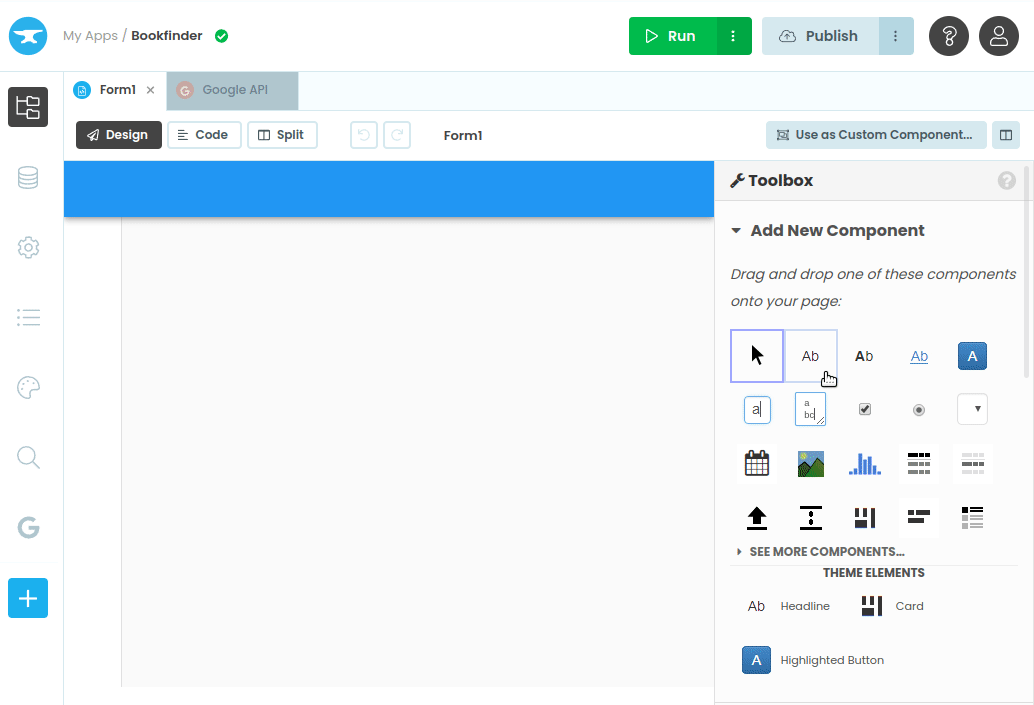
Give it the text ‘BookFinder’.
Step 3: Add author labels
Drag two Labels onto the Form in the Form Editor:
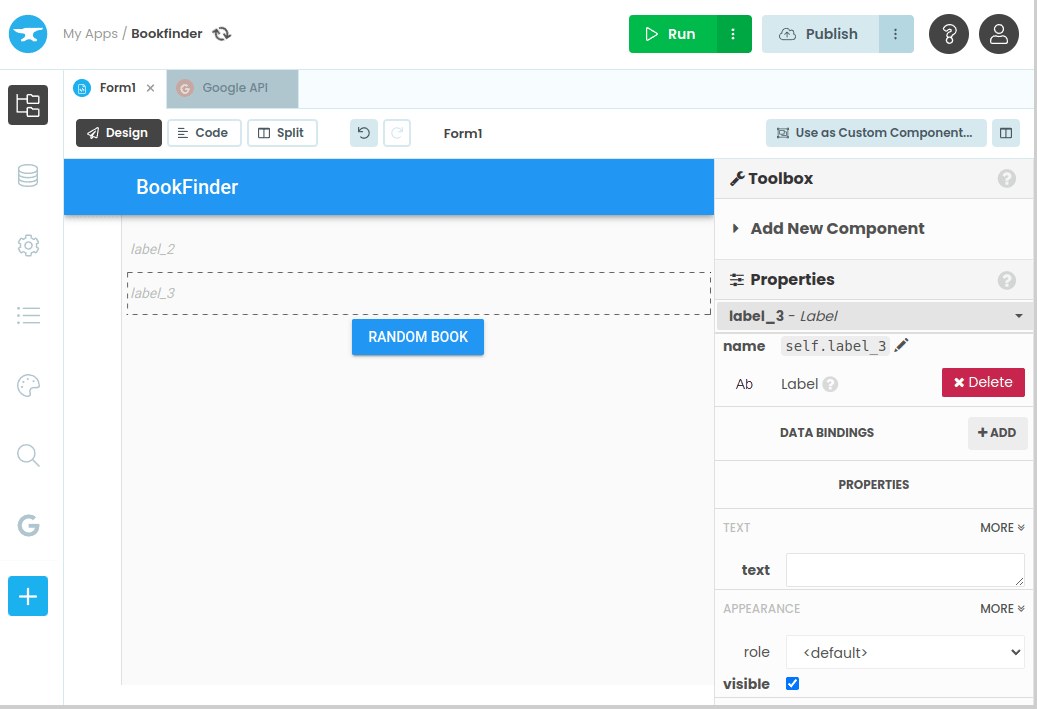
Give one the name book_title
and the other the name book_author
. In the text options, give them both the center
align and make book_title
bold:
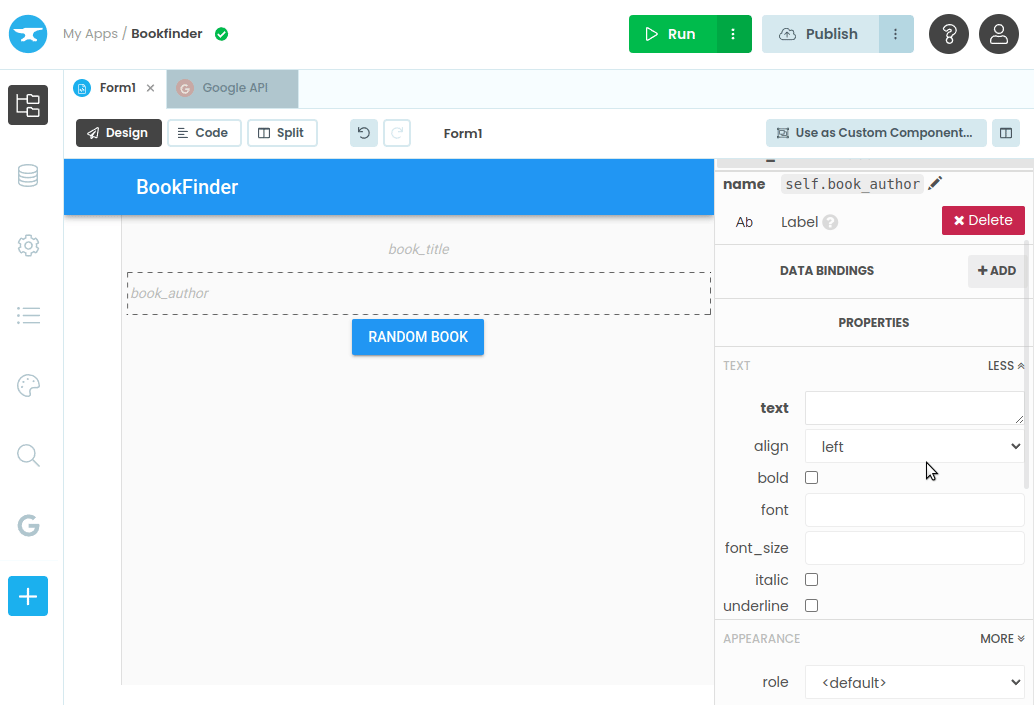
Now we have displayed book data from the Google Sheet at the press of a button. Nice job!
In Chapter 3, we’ll add some cover images from OpenLibrary.