Chapter 3:
Write client-side Python
Let’s now populate the DropDown we added in Chapter 2 with a list of category names from the Categories Data Table.
We’ll use Anvil’s search
query operator to do this.
Step 1: Run a Data Table search
Let’s switch to the Code View for our ArticleEdit Form.
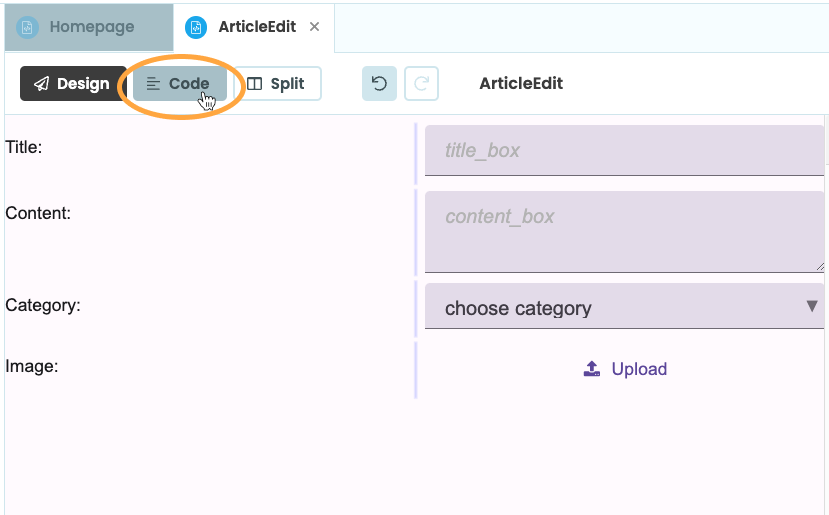
You will see some import statements and a Python class called ArticleEdit
that describes how the ArticleEdit Form behaves. Any code we write here will run on the user’s browser.
Let’s now search through the Categories Data Table and store the results in a variable. We can access any Data Table with app_tables.<data-table-name>
. Because we added Data Tables to our app, you should see the following import statement has automatically been added to the Form code:
from anvil.tables import app_tables
Add this to the __init__
function of the class:
self.categories = [
(cat['name'], cat) for cat in app_tables.categories.search()
]
This creates a list of 2-tuples of the form:
[
("First category name", <first_row_from_table>),
...,
("Final catgegory name", <final_row_from_table>)
]
The first element of each tuple is what will be displayed in the DropDown box. The second element of the tuple becomes the selected_value
property of the DropDown if that option is selected.
Step 3: Populate your dropdown
To display our list of categories in the DropDown, we need to set the items
property of our DropDown to the self.categories
list we just defined. Go back to your ‘ArticleEdit’ Form and add this line to the __init__
method:
# Any code you write here will run when the form opens.
self.category_box.items = self.categories
The ‘ArticleEdit’ Form should now look like this:
from anvil import *
import anvil.tables as tables
import anvil.tables.query as q
from anvil.tables import app_tables
class ArticleEdit(ArticleEditTemplate):
def __init__(self, **properties):
# Set Form properties and Data Bindings.
self.init_components(**properties)
# Any code you write here will run when the form opens.
self.categories = [(cat['name'], cat) for cat in app_tables.categories.search()]
self.category_box.items = self.categories
Step 4: Test that it works
To test what we’ve built so far, we can run our app and try it out. Let’s first set the ArticleEdit Form to be the Startup Form by opening up the App Browser, clicking the three dots menu next to ‘ArticleEdit’ and selecting ‘Set as Startup Form’. A lightning bolt icon will appear next to ArticleEdit to indicate that it is now the Startup Form
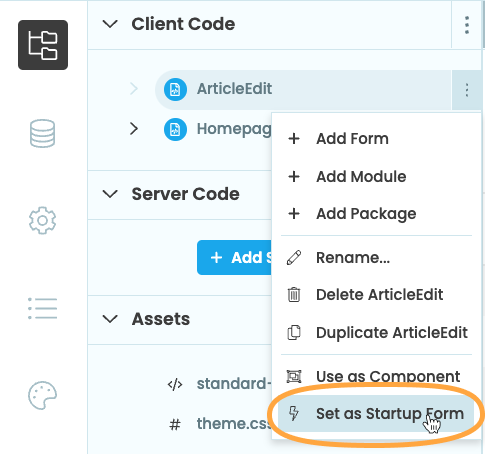
Now run the app by clicking the green Run button at the top of the Anvil Editor.

You should see the ArticleEdit Form running, and the dropdown should be populated with your categories:
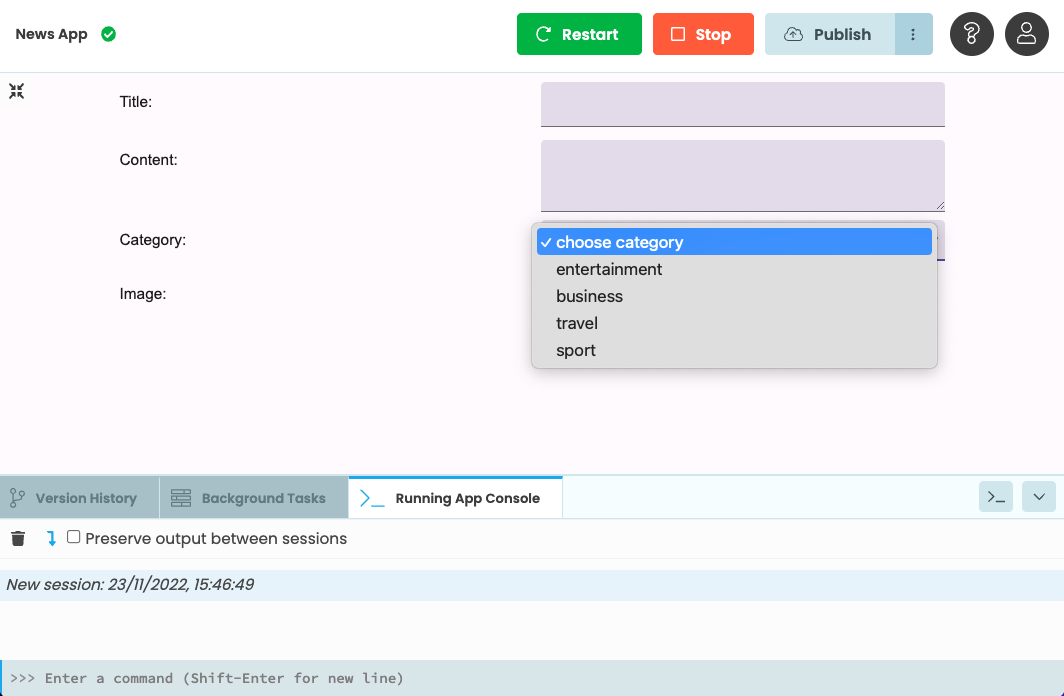
Remember to set Homepage back as the Startup Form when you’ve finished.
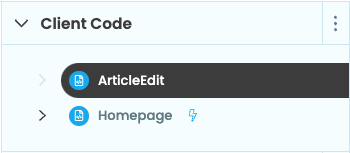
We’ve written the first piece of client-side Python code for our app. We’ve populated the DropDown on our ArticleEdit Form with categories from the Categories Data Table.
In Chapter 4, we’ll enable users to add articles to the database.