Chapter 5:
Make it real-time and update the location
Step 1: Add a Timer
We want to update our visualisations regularly to make sure that we’re getting the most recent data at any given time. We’ll do this by using a Timer component.
Go to Design view in the Form Editor and drop a Timer component onto the page. In the Properties Panel, set its interval
to 900 seconds (i.e., 15 minutes).
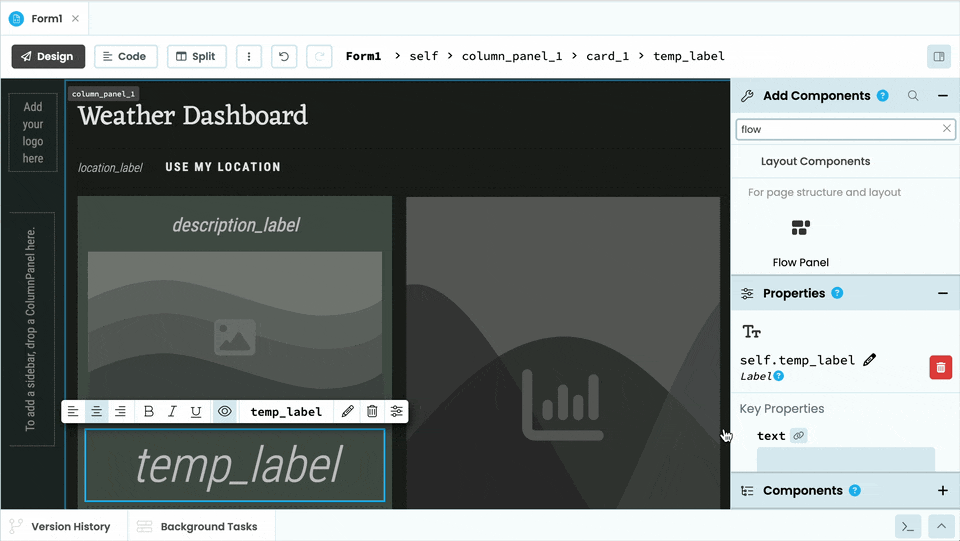
Scroll down to the bottom of the Properties Panel and click the blue arrows next to tick
to set up a tick
event handler.
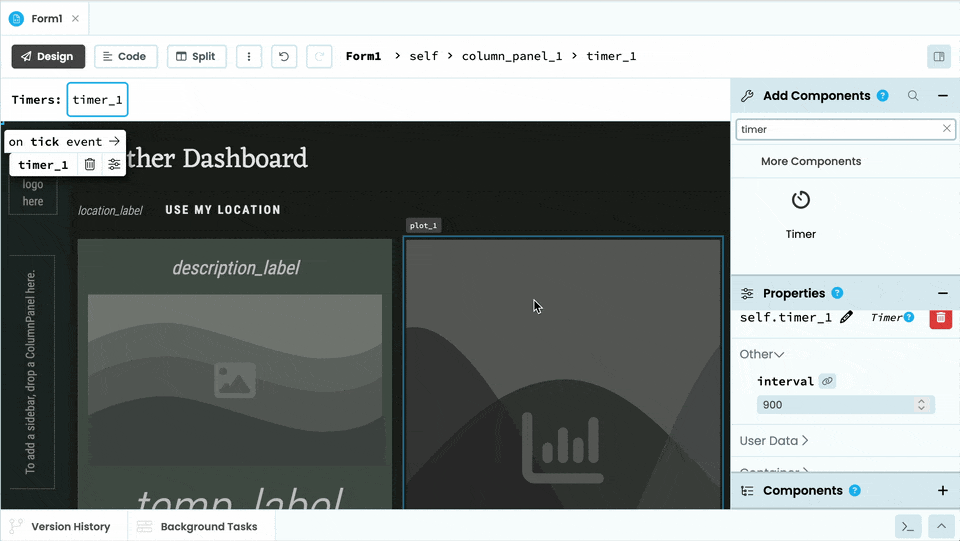
This will open the Split view and create a function for us. This function will be called once every 15 minutes (since that’s what we set for its interval
). Inside it, call refresh
. With this, our app will update every few minutes to show the latest weather data.
def timer_1_tick(self, **event_args):
"""This method is called Every [interval] seconds. Does not trigger if [interval] is 0."""
self.refresh()
Step 2: Get the user's current location
We’re now going to make it possible for the user to display the weather data from their current location. We’ll get the user’s location from their Navigator data, which we can access via the JavaScript window.navigator object. Anvil makes it easy to access any JavaScript object from your Python code by using anvil.js
.
anvil.js
will allow you to access all those low-level details from Anvil.Start by adding the following import statement to the top of your Form1 code:
from anvil.js.window import navigator
Enter the Design view and double-click on the location_button
that we added in Chapter 1. This will create an event handler for us. Inside the function, write the following code:
def location_button_click(self, **event_args):
"""This method is called when the button is clicked"""
navigator.geolocation.getCurrentPosition(lambda p: self.set_location(p.coords.latitude, p.coords.longitude))
self.location_button.enabled = False
We’re also disabling the button after clicking it, since it takes a while for the app to refresh and display the user’s location. This will signal to users that something is happening even if the page hasn’t refreshed yet.
Run the app. Click ‘Use My Location’ and make sure everything is updating.
And that’s it! Our app is complete, we just need to publish it and share it with the rest of the world.