The haunted keyboard
Python gives you superpowers - you can even import antigravity
. Like all superpowers, it’s up to you whether you use
them for good or evil. Today we’re going to use them for evil.
Imagine your colleague is thirsty. They leave their desk and go to make a cup of tea, taking the company branded tea tray with them.
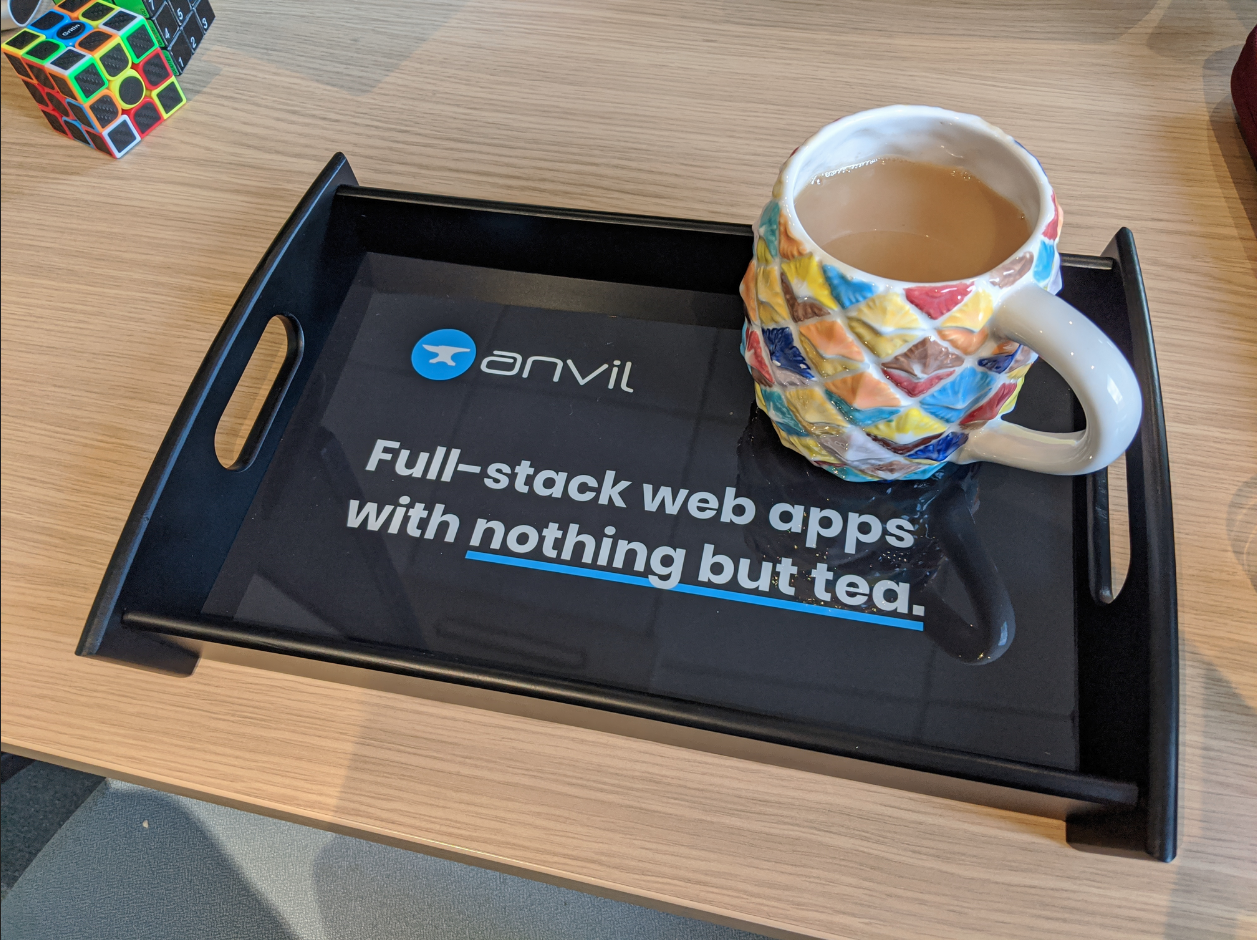
They make an elementary mistake. They forget to lock their screen. You have access to their machine and you’re going to make the most of it. What can you do with Python to inflict the maximum confusion without getting fired?
The pynput
library allows you to take control of keyboard input from Python. You can listen to keystrokes as they
are entered, and inject your own keystrokes into the input stream.
So you open a terminal window and pip install pynput
. Typing furiously, you write a script to randomly hold down
the Shift key:
from pynput.keyboard import Controller
keyboard = Controller()
from random import randint
from time import sleep
if __name__ == "__main__":
while True:
with keyboard.pressed(Key.shift):
sleep(randint(2, 5))
sleep(randint(2, 5))
You run it in the background and hurriedly close the terminal window:
$ python3 perfectly_normal_script.py &
Your colleague is returning. You rush back to your seat and wait for the confused exclamations from the other side of the desk:
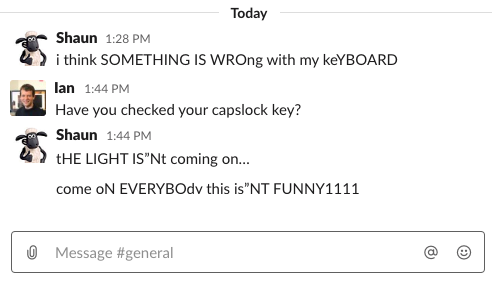
Helpful.
They turn their machine off and on again and everything is fine. “Must have been a glitch,” they think to themselves. They go about their day none the wiser.
The browser in Python
Sooner or later, the call of tea comes again. They leave their desk, and again their screen is unlocked. Time for another prank!
Fool me twice… shame… shame on…
Won’t get fooled again.
George W Bush
President, United States (2001-2009)
If you’re reading this you’ve probably heard of Python in the browser. But did you
know that the reverse is true as well? You can get the browser in Python!
There’s a built-in module in the standard library called webbrowser
that can open your default web browser, among
other similar things.
So you make them visit your favourite website every time they hit the Shift key. This time you use pynput
to listen
to keystrokes and repond when Shift is pressed:
import webbrowser
from pynput import keyboard
def on_press(key):
if key == keyboard.Key.shift:
webbrowser.open_new('https://anvil.works')
if __name__ == "__main__":
with keyboard.Listener(on_press=on_press) as listener:
listener.join()
You’re not going to make it so easy for them this time. You run it in the background again, but you also make sure it runs every time their computer starts:
$ echo 'python3 /home/shaun/.the_browser_in_python.py &' > /etc/rc.local
$ chmod +x /etc/rc.local
Your colleague returns. The confused exclamations begin once more:
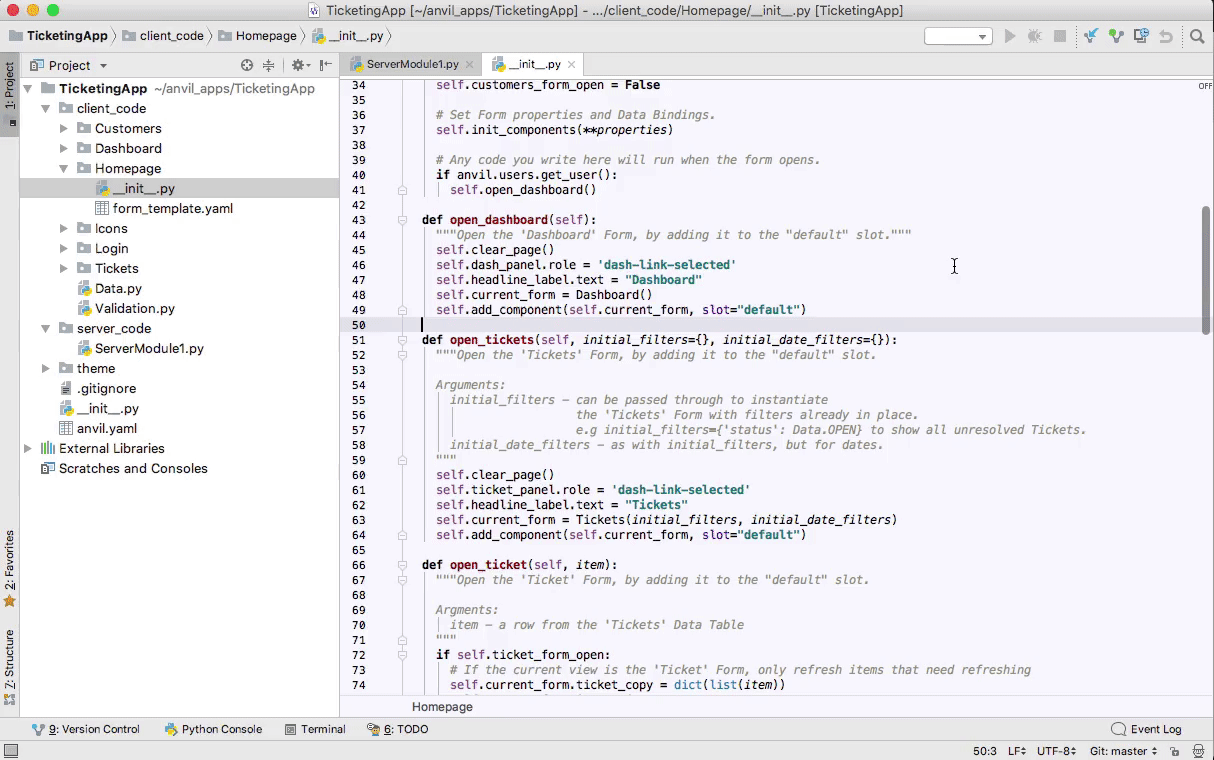
You can’t write much code without pressing Shift.
There’s a lesson here
You may think you’re being a troll, but you’re inadvertently teaching your colleagues a valuable engineering skill - Root Cause Analysis (RCA).
Your colleague initially dismissed the issue as a glitch and restarted their computer. They identified the apparent problem: when they type, characters cOME OUT IN UPPer case at seemingLY RANDom intervaLS.
Because they’re a nerd, they started making a diagram:

I feel a diagram coming on…
They immediately came up with a proximal cause. They guessed, correctly, that there must be something temporarily wrong with their input system, so they restarted their computer to see if that would fix it. Problem solved! Time to update the diagram:
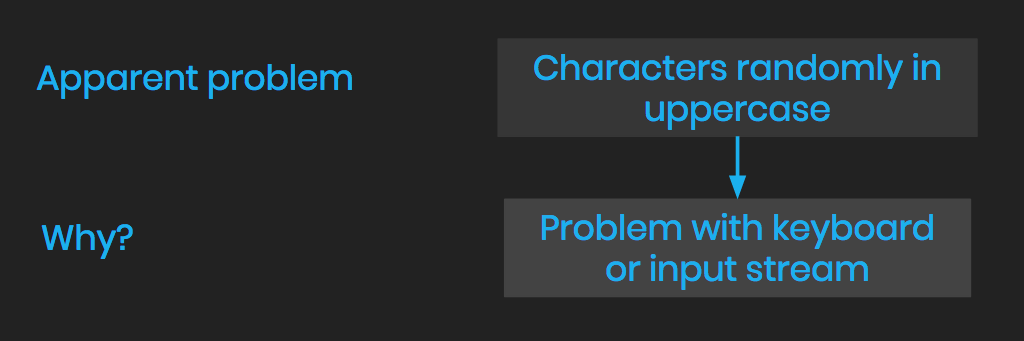
But another apparent problem appeared, and it clearly wasn’t a temporary keyboard input glitch. Now the diagram has a dangling thread:
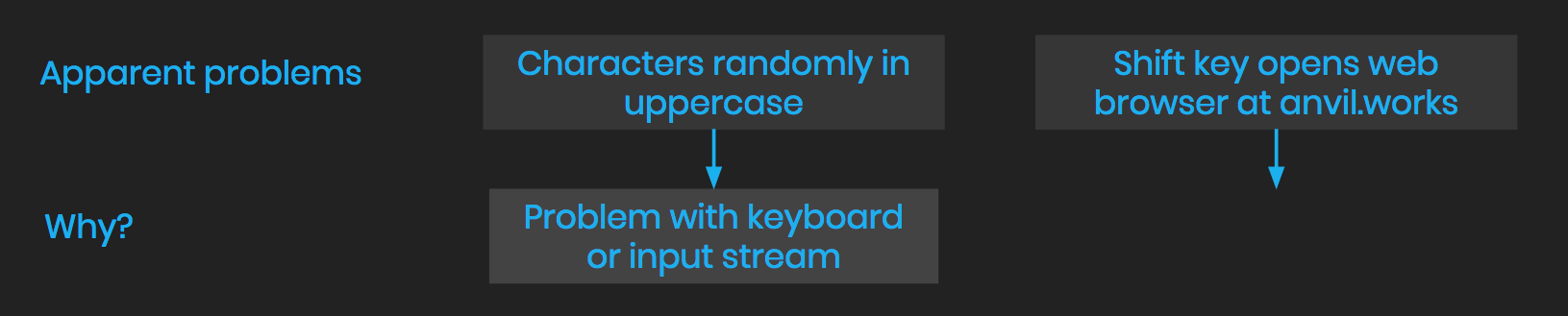
They did some investigation, and checked their /etc/rc*
files, because the problem was surviving a reset: ah hah!
There’s a script here that has clearly been created by a trollish colleague.
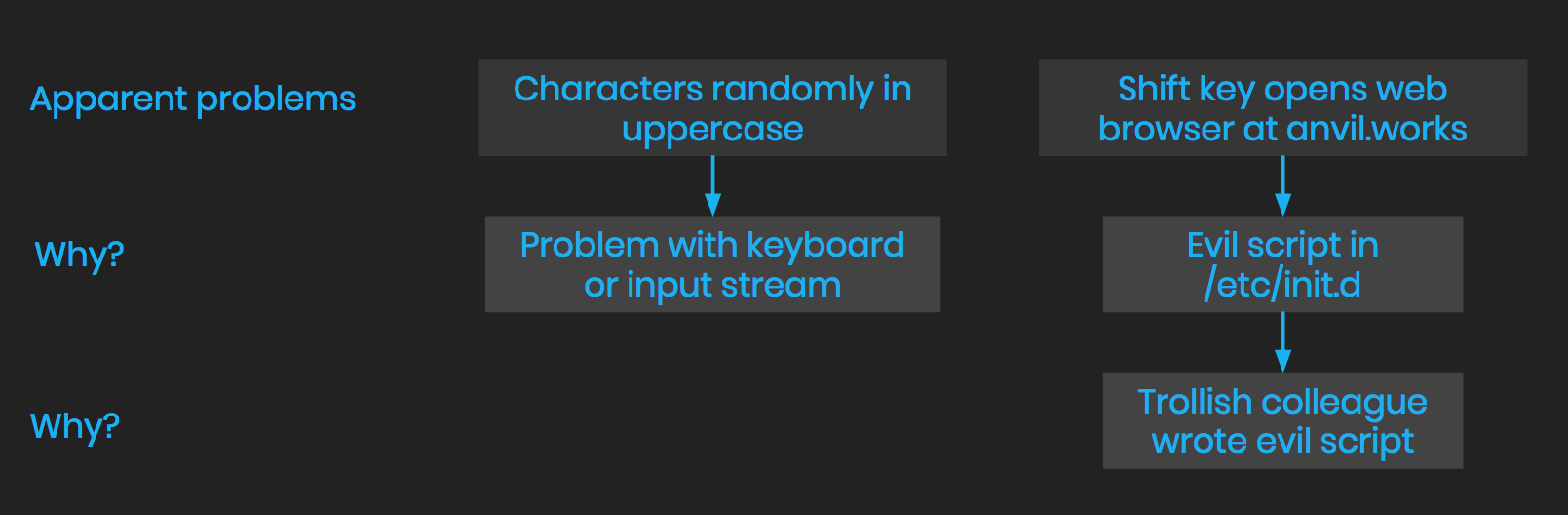
It’s all starting to come together. Perhaps the other apparent problem was also caused by a nefarious troll script.
They grep their entire filesystem for pynput
and find the original perfectly_normal_script.py
that you ran in the background.
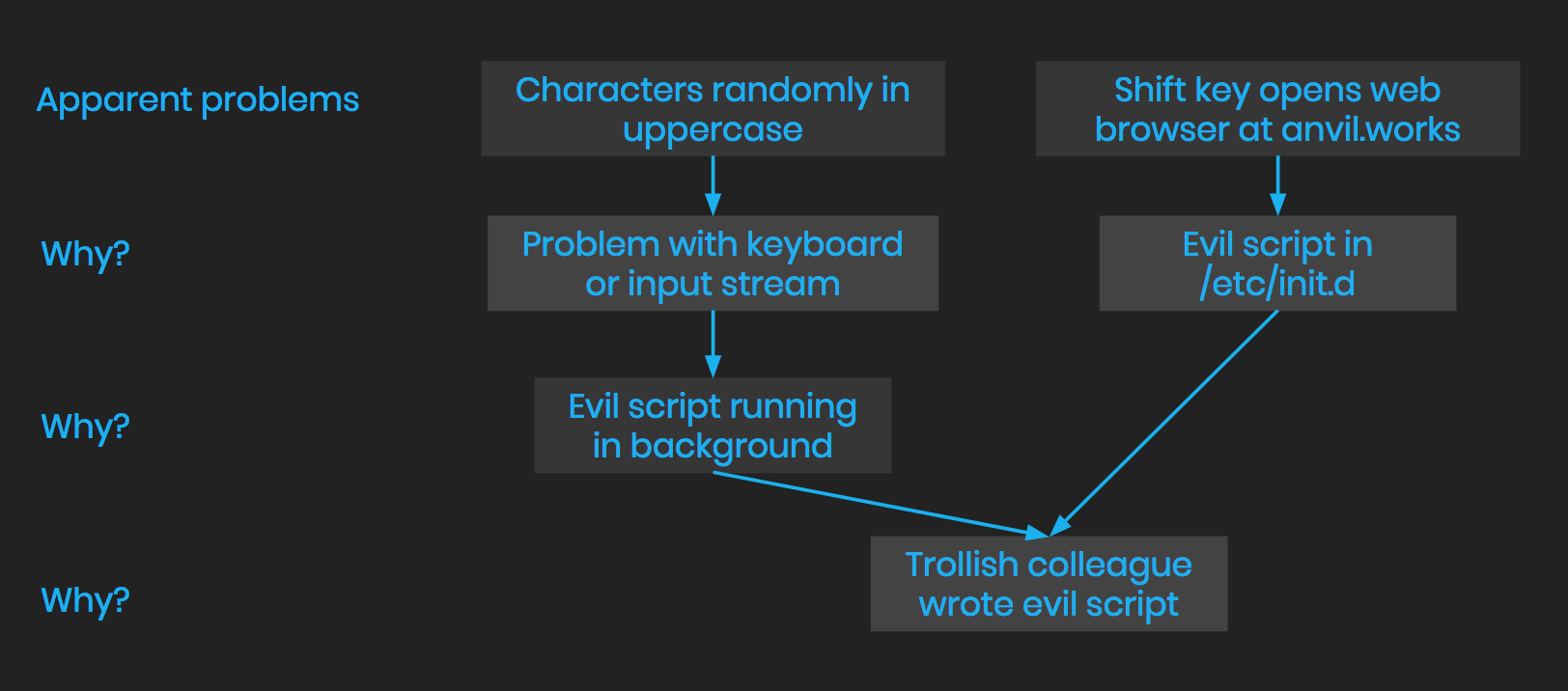
But these scripts are not the root cause - they must have come from somewhere, so your colleague asks themselves another ‘why?’ question. Why are these scripts on my system? The answers are obvious:
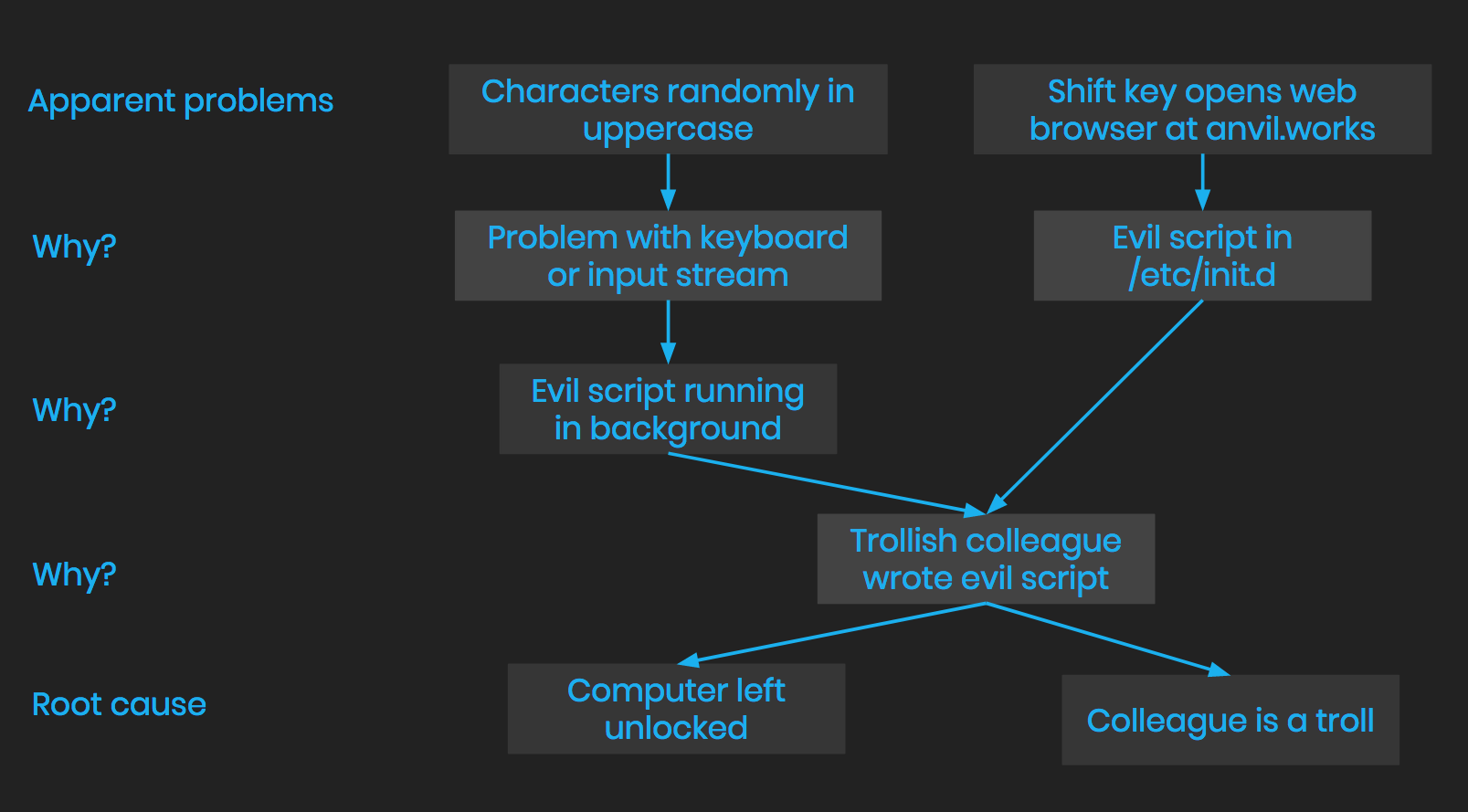
The questions could go on.
- Why did I leave my screen unlocked?
- Why is my colleague such a troll?
- Is it to do with his upbringing or is there something in his genes that leads to such unhelpful behaviour?
- What particular genes are involved in this kind of attention seeking?
- How did those genes evolve? What were the evolutionary pressures?
But these are not things your colleague can act upon and fix. They’ve reached the final level of explanation that they have control over - these are the things we honour with the name root cause. They resolve to never leave their screen unlocked again, and the company’s data security is vastly improved. You’ve done them a favour, which of course is what you meant to do all along.
Well done! A productive day’s work. Now treat yourself to a cup of tea.
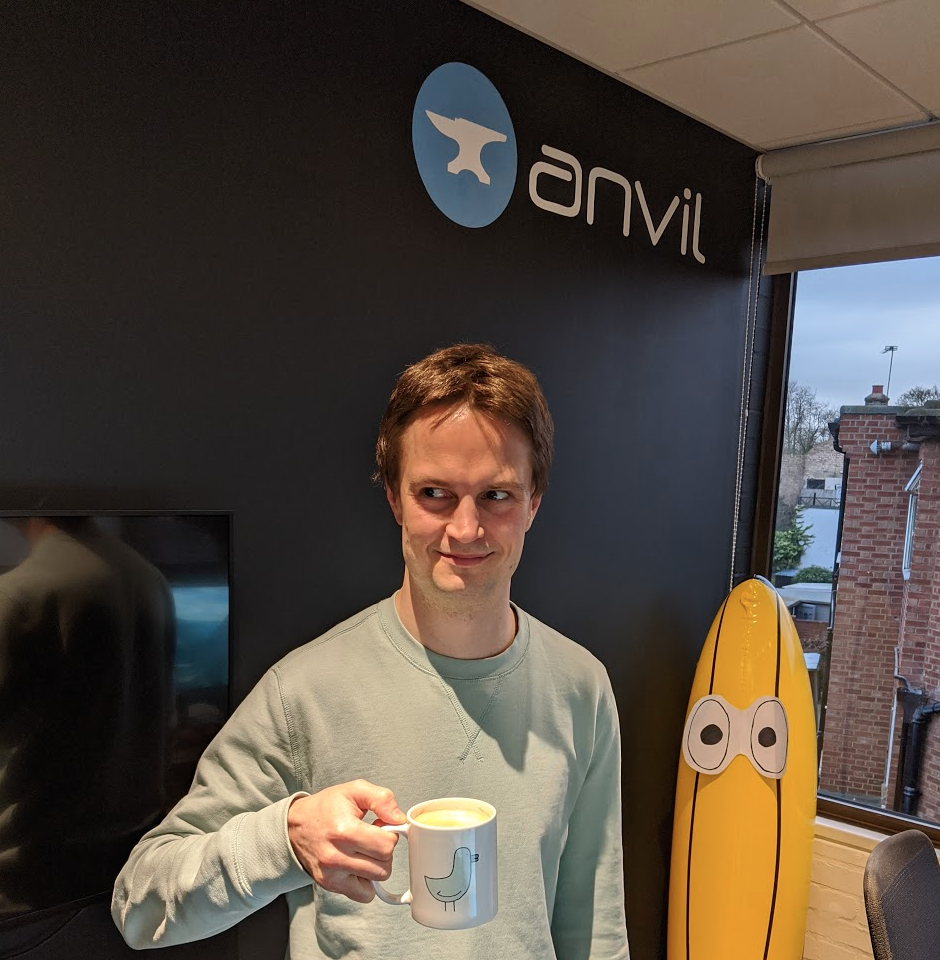
Shaun’s “innocent” face needs some work.