Day 7 of the Anvil Advent Calendar
Build a web app every day until Christmas, with nothing but Python!
Decorate a Virtual Cookie
One of my favorite things to do during the holidays is bake lots of Christmas cookies! For Day 7 of our Advent Calendar, I built a web app using the Canvas API to decorate mess-free, no-bake virtual Christmas cookies!
Check out my app and decorate your cookies by following the link below. And keep reading to see how I built the app.
https://decorate-a-christmas-cookie.anvil.app
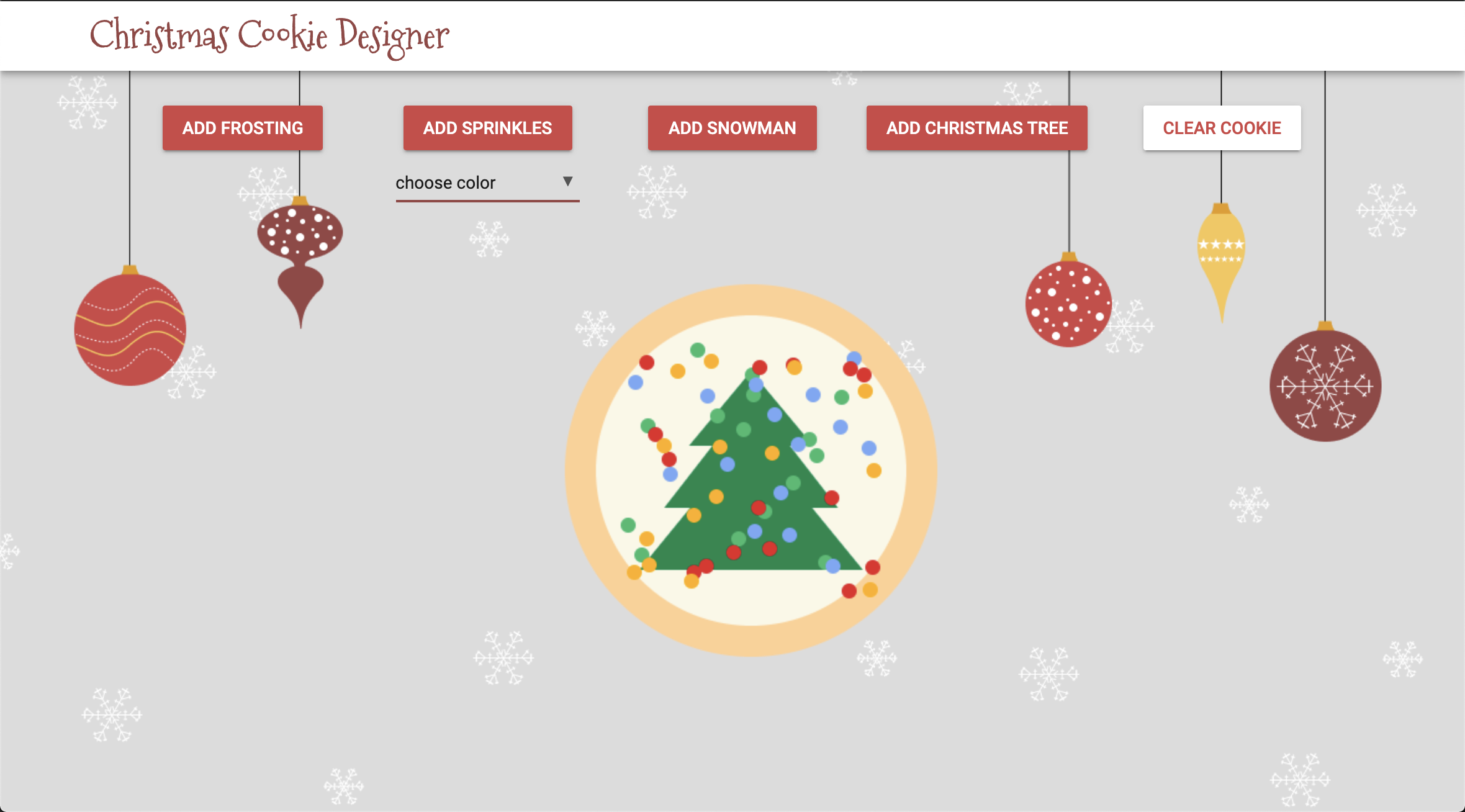
Making Lots of Circles
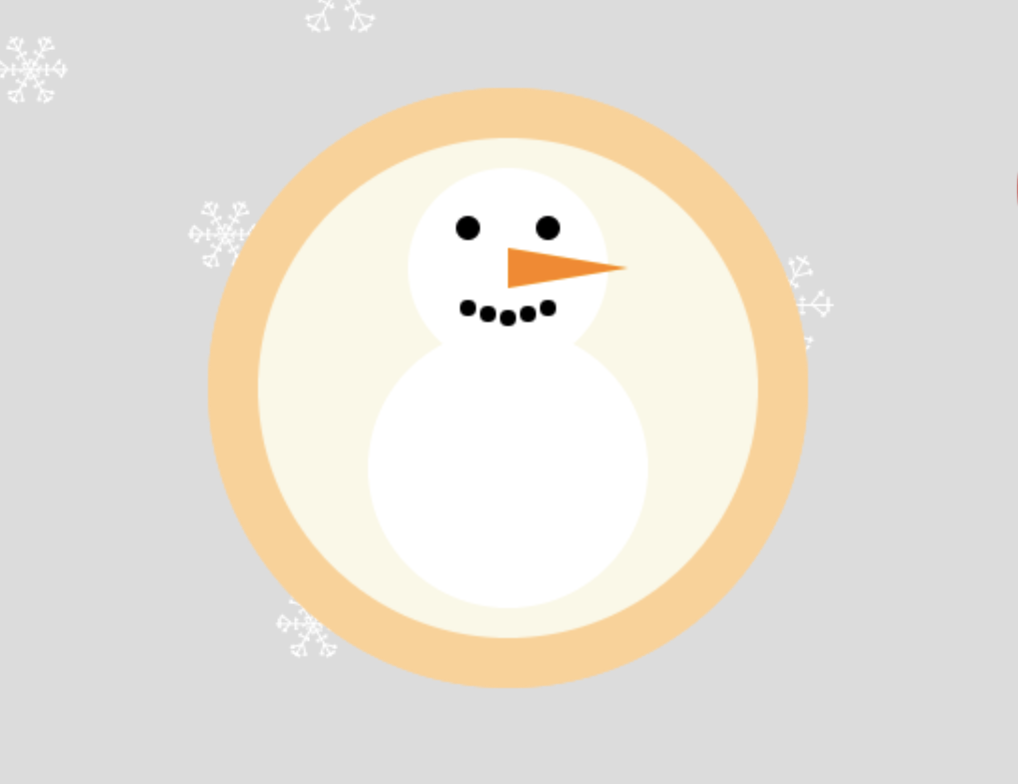
I made a simple cookie decorator using the Canvas component by just building circles and triangles of different sizes and colors. By adding more elements, the app could easily be extended to add more options or be more complicated.
Because my app makes extensive use of circles, I first defined a function to draw a circle. The function takes in the desired x and y coordinates of the circle and its radius as arguments:
def draw_circle(self, x, y, radius):
c = self.canvas_1
c.begin_path()
c.arc(x, y, radius, 0, 2 * math.pi)
c.close_path()
This function is called when the form opens to create the cookie base. It’s also used to make the frosting, sprinkles and snowman.
Adding Random Sprinkles
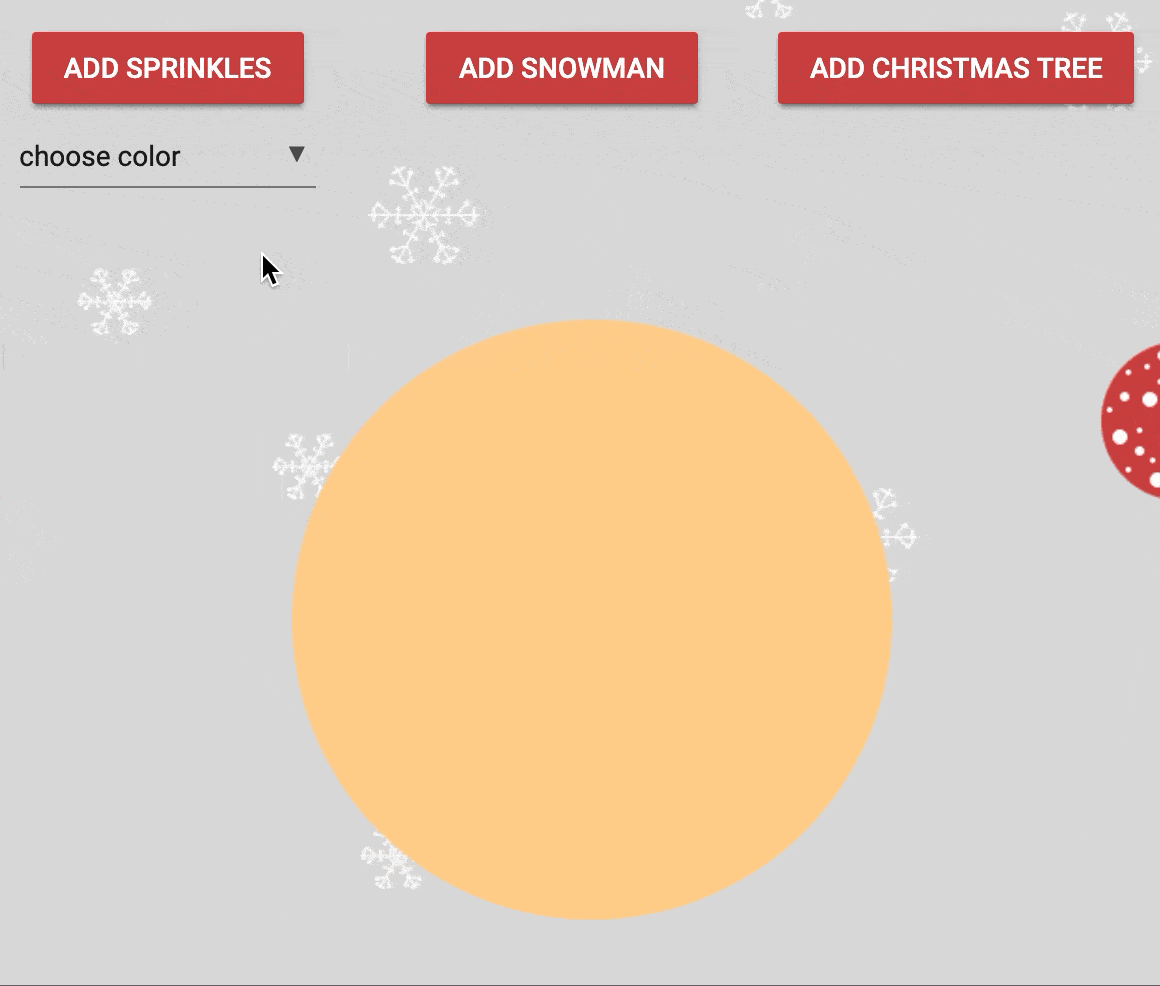
The x and y coordinates for the sprinkles are chosen randomly each time the sprinkles_button
is clicked:
import random
...
def sprinkles_button_click(self, **event_args):
"""This method is called when the button is clicked"""
color = self.color_dropdown.selected_value
if color is None:
color = "#e62325" #defaults to red
self.canvas_1.fill_style = color
x_list = list(range(400, 600))
x_list = random.sample(x_list, 15)
y_list = list(range(100, 300))
y_list = random.sample(y_list, 15)
for x,y in zip(x_list, y_list):
self.draw_circle(x, y, 6)
self.canvas_1.fill()
Christmas Tree Triangles
For the Christmas tree, I just made three overlapping green triangles that get progressively smaller as they move up the y-axis. The following code draws one triangle:
c = self.canvas_1
c.fill_style = '#00884b'
c.begin_path()
c.move_to(410,280)
c.line_to(590,280)
c.line_to(500,170)
c.close_path()
c.fill()
You can check out the entire source code by cloning the app:
Give the Gift of Python
Share this post: