Day 16 of the Anvil Advent Calendar
Build a web app every day until Christmas, with nothing but Python!
Earlier this month, we built an app where you can log in and create a Christmas wishlist, and we even added a couple of extensions to it (See day 7 and day 13 ). Now, we are going to make it possible to access your wishlist by email and curl, with just a few lines of code!
Creating an HTTP Endpoint
Let’s first add a function to our Server Module so we can connect to the given URL. Because we just want to use this to read our data, we will make this a ‘GET’ request. You can learn more about HTTP APIs by checking out our tutorial.
@anvil.server.http_endpoint("/GetList", methods=['GET'])
def get_list_http():
gifts = app_tables.gifts.search()
wish_list = [{"name": giftData['description'], "claimed_by": giftData['claimed_by']} for giftData in gifts]
return wish_list
Emailing the Wishlist
We’ll add another function to the server side, which will allow us to send our emails, by using the anvil.email module.
# This function requires us to be logged in to be used
@anvil.server.callable(require_user=True)
def email_list(email):
rows = app_tables.gifts.search()
body = ''
for i,row in enumerate(rows):
body += f"<h2>{row['description']}</h2>"
if row['claimed_by'] is not None:
body += f"<div>Claimed by {row['claimed_by']}</div>"
body += f'<img width=200 src="cid:{i}"/>'
anvil.email.send(
to=email,
from_address=anvil.users.get_user()['email'],
from_name="My Wish List",
subject="Here is my Christmas Wish List",
html = body,
inline_attachments = {str(i):row['photo'] for i,row in enumerate(rows)}
)
Now, let’s add a button to our main page that displays an alert where we can enter the email address we want the list sent to.
def email_button_click(self, **event_args):
email_data = {}
if alert(content=EmailDetails(item=email_data), large=True, buttons=[('Send', True), ('Cancel', False)]):
anvil.server.call('email_list', email_data['email'])
Finally, we’ll make the button visible only when we’re logged in by adding data bindings.
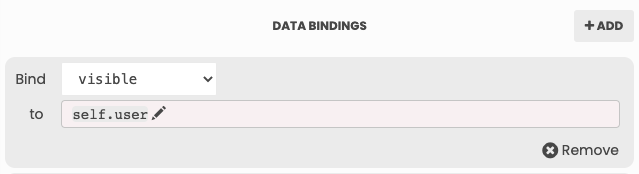
And that’s it! Now we can receive an email and take our wishlist on the go, or simply send it to a friend so they can view it easily.
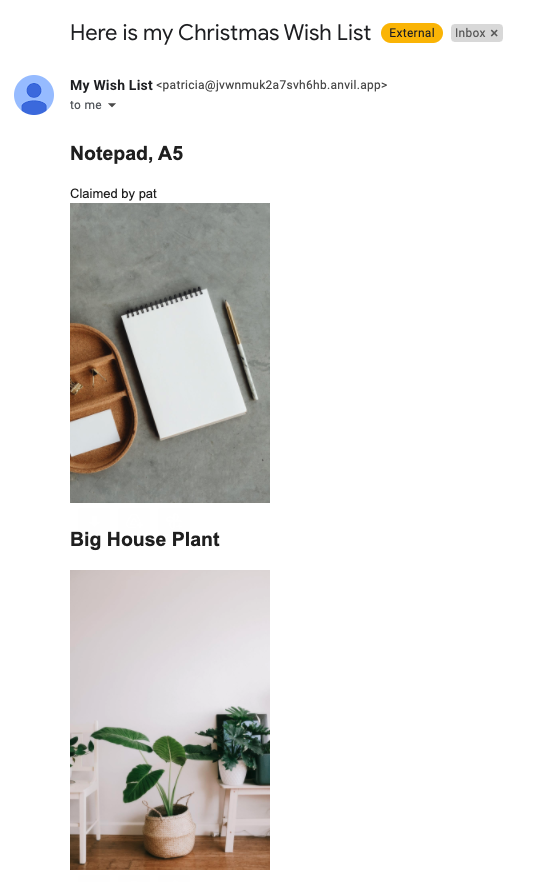
You can look at the full source code of the app with all the extensions by cloning the app in the link down below.
Give the Gift of Python
Share this post: