User Interfaces
With Anvil, you can build the user interface of your web app in Python: either by using Anvil’s drag-and-drop designer, or by writing code.
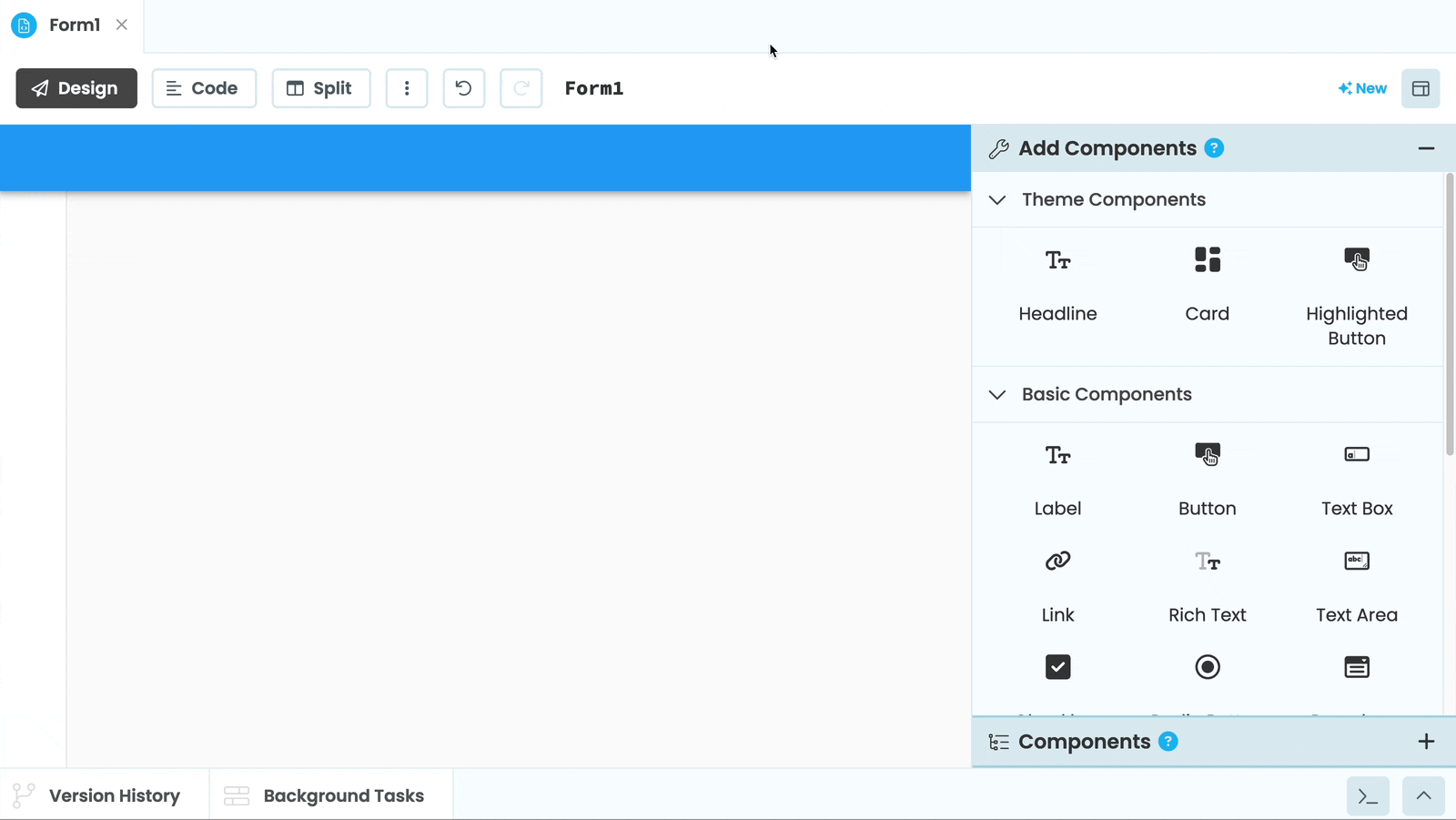
Dragging and dropping components to make the UI of an Anvil app
You can then bring your app to life by writing Python code that runs in the browser. Check the Quickstart for an introduction.
Structure of an Anvil UI
Your app’s user interface is made up of one or more Forms, which are pages (or parts of pages) that you create in the Anvil Editor.
Your Forms are made up of components such as buttons, text boxes, panels, and so on. In fact, your Forms are themselves components!
You can even define your own components and have them appear in the toolbox of the visual designer.
Python Code
Each Form is also a Python class, with Python code that runs in the web browser when a user opens your app. You can specify code to run when events fire (for example, when the user clicks a particular button). Your code can control the components on your Form, to dynamically display data, navigate around the app, and generally control a dynamic UI.
And, of course, you can make calls to your app’s Server Modules, which run on the Anvil server instead of in the browser, and to Python code outside of Anvil.
Do you still have questions?
Our Community Forum is full of helpful information and Anvil experts.