Setting up the Uplink
To set up the Uplink, you need to enable it in the Anvil IDE, pip install
a Python library, then write three lines of
code.
Enabling the Uplink
The Anvil uplink is a library you add to your own code, running outside Anvil. It connects securely to the Anvil server, and allows your Anvil app to call functions in your project. You can also call server modules within your app from your uplinked code. The Anvil uplink works through most firewalls, as it initiates the connection to the Anvil server.
Start by adding the Uplink to your app, by clicking the + button in the sidebar menu, and choosing Uplink:
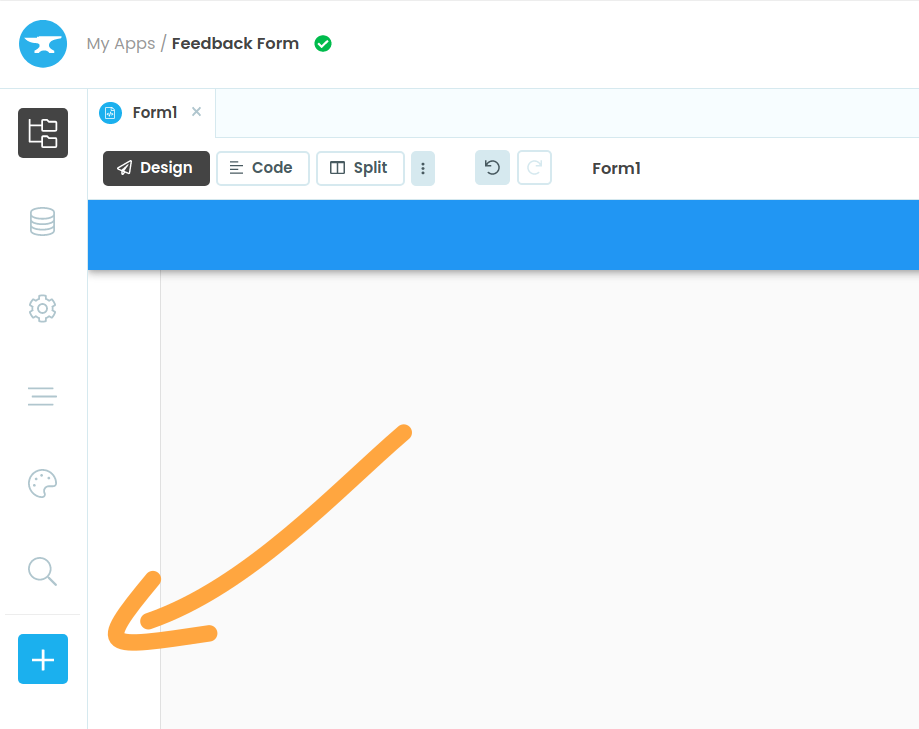
Click the + button, then select Uplink
If you haven’t already deployed your app, you’ll see a button labelled Add Uplink keys. You’ll need to click it before moving on to the next step:

If you’ve already deployed your app, you’ll see all your deployment environments.
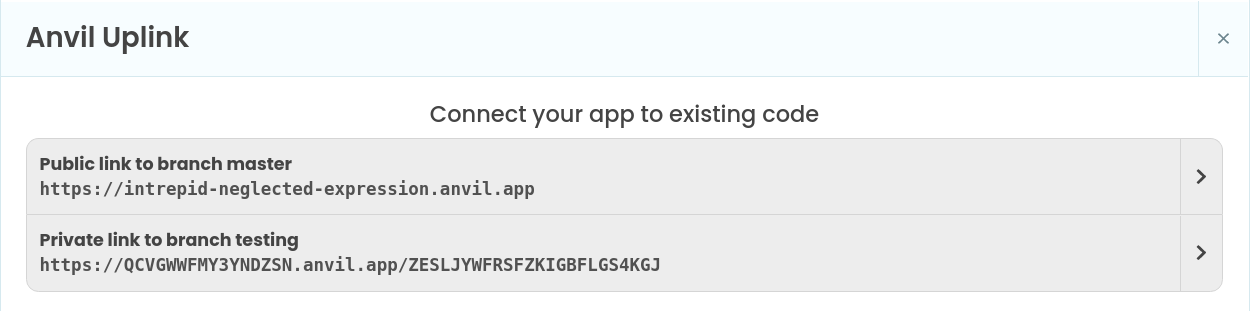
Click one to select it, and click Enable next to Server Uplink Key or Client Uplink Key (learn about the difference). This will create a secret key that you can use to connect external code to your application:
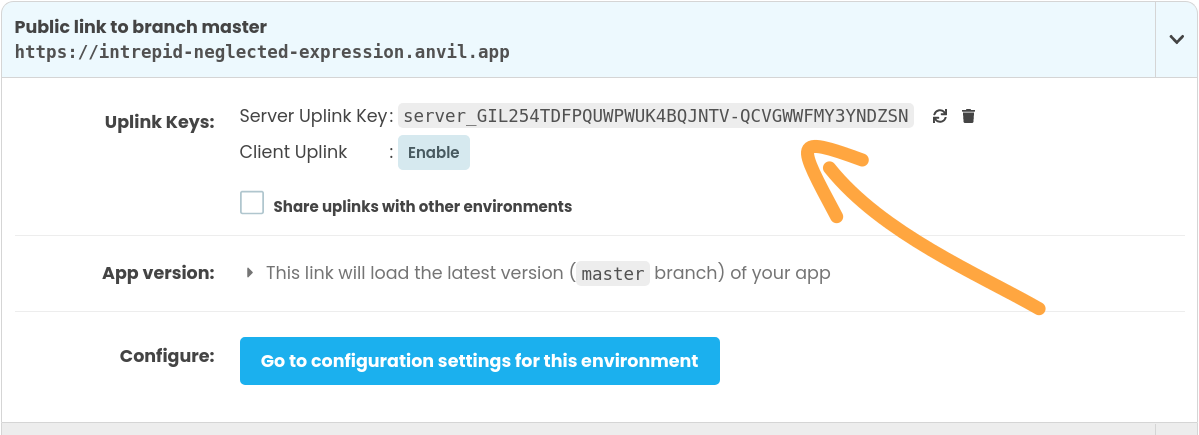
Connecting
In your local Python code, call anvil.server.connect()
with your app’s connection key in order to link this program with your Anvil app. This establishes a connection in a background thread and will keep attempting to reconnect to Anvil if it fails (for instance, if you lose your local internet access temporarily).
# In a script on your own machine (or anywhere)
import anvil.server
anvil.server.connect("[YOUR UPLINK KEY]")
@anvil.server.callable
def get_file():
# Return a file from this local machine
return anvil.media.from_file("./image.jpg", "image/jpeg")
anvil.server.wait_forever()
The function anvil.server.wait_forever()
is just a useful shortcut to keep your Python script running, to allow your app to anvil.server.call
functions in it.
You can use any other way to keep the process alive - for instance,
anvil.server.connect("[YOUR UPLINK KEY]")
@anvil.server.callable
def get_file():
return anvil.media.from_file("./image.jpg", "image/jpeg")
while True:
# keep the process alive to respond
sleep(1)
You can even run the Uplink in a Python REPL!
Disconnecting
Sometimes you may only want to connect your code temporarily using the Anvil Uplink. To safely close down an Uplink connection, just call anvil.server.disconnect()
when you are ready to end the connection.
import anvil.server
def open_anvil_connection(uplink_key):
anvil.server.connect(uplink_key)
do_something_cool_using_uplink()
anvil.server.disconnect()
This can be done within a context manager. For example:
import anvil.server
from contextlib import contextmanager
@contextmanager
def open_anvil_connection(uplink_key):
anvil.server.connect(uplink_key)
yield
anvil.server.disconnect()
You can then use it in a with
statement which politely closes down the connection when it’s done:
with open_anvil_connection("[YOUR UPLINK KEY]"):
data = anvil.server.call("some_remote_function")
results = perform_local_calculation(data)
anvil.server.call("write_back_crunched_data", results)
Advanced setup
Custom setup code
Sometimes, it is necessary to initialise an uplink’s session before interacting with your app. For example, a client (unprivileged) uplink process might need to authenticate with the app before it will be allowed to perform certain operations.
If you pass the init_session=
keyword parameter to anvil.server.connect
, it will be called after the uplink connection is established, but before any other interaction (such as server calls or function registrations) have occurred. If connection is lost and the uplink library reconnects, init_session
will be called again before any interaction occurs. This guarantees that init_session
has completed whenever you interact with the Anvil app.
import anvil.users
def setup():
anvil.users.login_with_email("my_user@example.com", "MY_PASSWORD")
anvil.server.connect("[YOUR UPLINK KEY]", init_session=setup)
anvil.server.call("some_func")
If init_session
raises an exception, all subsequent Anvil interactions will fail and raise exceptions.
Suppressing connection messages
By default, the Uplink prints some output during the connection phase:
Connecting to wss://anvil.works/uplink
Anvil websocket open
Authenticated OK
You can pass an optional keyword argument “quiet
” to anvil.server.connect()
to suppress this. Errors (such as connection failure and reconnection attempts) will still be printed.
# Connect without printing to console
anvil.server.connect("[YOUR UPLINK KEY]", quiet=True)
Do you still have questions?
Our Community Forum is full of helpful information and Anvil experts.