Accelerated Tables
In May 2022, Anvil announced a beta release of Accelerated Tables. This is a new back-end architecture for Data Tables, bringing increased speed, scalability and flexibility. The Data Tables API does not change, but as this is a major change we are rolling this out slowly. Accelerated Tables is currently an opt-in beta program.
Enabling the Accelerated Tables Beta
In order to enable Accelerated Tables for your app, open Settings, then choose Data Tables, then check the Accelerated Tables option. You do not need to change your app’s code.
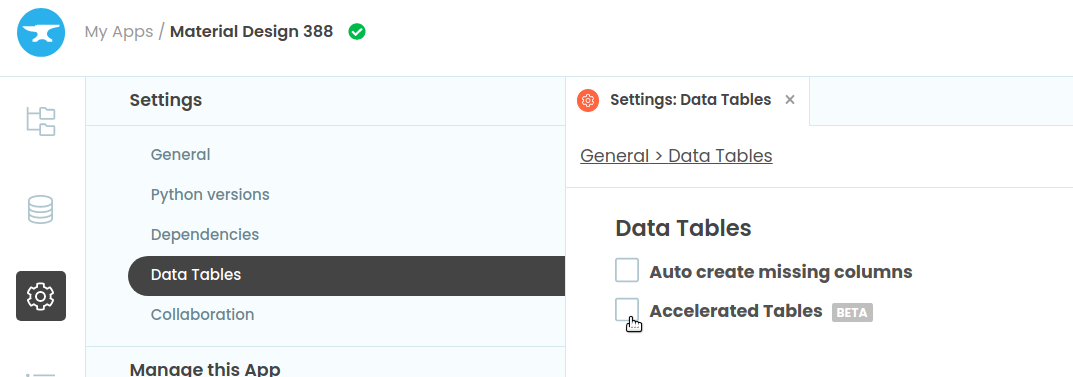
Click here to enable Accelerated Tables
Testing safely
The switch to enable Accelerated Tables is stored as a configuration option in your app’s source code. This means that if you have published a version of your app, you can safely experiment with the development version of your application without affecting production users.
Data written using Accelerated Tables can be read by the original Data Tables implementation, and vice versa, so it is safe to switch this option on and off.
New Features
Accelerated Tables improves speed for most access to your Data Tables. It also gives you extra benefits and control over:
Linked rows
Loading linked rows is significantly faster when using Accelerated Tables, particularly “Link to Multiple” rows. If you have previously avoided data architectures with these links, now might be the time to try them again.
Column-restricted views
With Accelerated Tables, you can control which columns are accessible from a view, by passing q.only_cols()
. For example, the following code will return a view of the users
table that contains only the email
and enabled
columns:
@anvil.server.callable
def get_users():
return app_tables.users.client_readable(q.only_cols("email", "enabled"))
Batch add, update and delete rows
Adding, updating and deleting multiple rows in your Data Tables is now a lot faster. You can now add, update and delete rows in batches, saving round trips to the database.
To add multiple rows to a Data Table at once, you can pass a list of dictionaries to add_rows()
:
app_tables.my_table.add_rows([
{'name': 'Ada', 'age': 36},
{'name': 'Katherine', 'age': 101}
])
Use the anvil.tables.batch_update
context handler to update rows in batches:
ada_row = app_tables.my_table.get(name='Ada')
katherine_row = app_tables.my_table.get(name='Katherine')
with anvil.tables.batch_update:
ada_row['name'] = 'Ada Lovelace'
katherine_row['name'] = 'Katherine Johnson'
To delete multiple rows at once, you can use delete_all_rows()
or the anvil.tables.batch_delete
context handler:
#using the context handler
rows = app_tables.my_table.search(name=None)
with anvil.tables.batch_delete:
for row in rows:
row.delete()
#using delete_all_rows()
app_tables.my_table.search(name=None).delete_all_rows()
Explicit cache control
With Accelerated Tables, you can control which columns are loaded from a table search, using the new fetch_only
search modifier.
For example, if you are querying the users
table, but only need the values of the "email"
and "enabled"
columns, you can write:
from anvil.tables import query as q
rows = app_tables.users.search(q.fetch_only("email", "enabled"))
This will speed up the query, by only fetching the data you need. It is possible to access other columns in rows returned from this search, but it requires a round-trip to fetch that data from the database.
You can also control what data is fetched from linked rows, by using nested fetch_only
specifications. For example, if your users
table had a link column called group
, and you only want the "name"
column from those columns, you can write:
rows = app_tables.users.search(q.fetch_only("email", group=q.fetch_only("name")))
Other changes
List available tables
from anvil.tables import app_tables
table_names = list(app_tables)
Access a table using subscript notation
my_table = app_tables["my_table"]
Feedback
All code that works with Data Tables should work with Accelerated Tables. If you’re experiencing an issue, please post in the Anvil Community Forum with a description of the problem.
Do you still have questions?
Our Community Forum is full of helpful information and Anvil experts.