Quickstart: JavaScript
Learn how to drive JavaScript functions and external libraries from Anvil.
Follow this Quickstart guide to create an app that drives a Quill text editor (all from Python!).
Include the External Library
Check the library for the installation options. There will often be a CDN option with some HTML. When you include the HTML in your project, the library will be pulled in from an external host.
Find this code and paste it into your native-libraries.
<!-- Main Quill library -->
<script src="//cdn.quilljs.com/1.3.6/quill.js"></script>
<!-- Theme included stylesheets -->
<link href="//cdn.quilljs.com/1.3.6/quill.snow.css" rel="stylesheet">
Import JavaScript into Anvil
The anvil.js
module gives you access to the window object.
Any JavaScript object from the window can be imported into Anvil.
We need the Quill
constructor from JavaScript so import it.
from anvil.js.window import Quill
# import the Quill constructor from JavaScript
Convert JavaScript to Python
Start using the library. This involves reading the documentation and adapting examples to work in Anvil. Below is some JavaScript from the Quill documentation.
// JavaScript code from Quill documentation
var quill = new Quill('#editor', {
modules: { toolbar: true },
theme: 'snow'
});
quill.on('text-change', function(delta, oldDelta, source) {
console.log(source, quill.getText());
});
Make it Python!
- dictionary string keys must use quotes in Python
true
andfalse
becomeTrue
andFalse
null
becomesNone
- Inline functions with multiple lines of code should be extracted into separate functions
Add a ColumnPanel in the design view. We’ll tell Quill to use the ColumnPanel as the editor with anvil.js.get_dom_node()
.
Call the Quill
constructor inside your Form’s __init__
method with the DOM node and some options.
We’ll also add a method text_change()
to handle the Quill ’text-change’ event.
__init__
method runs before the Form, and its components, are added to the page. Some JavaScript functions will require DOM nodes to be on the page in order to execute correctly. If this is the case, or if you notice some strange behaviour, try moving the JavaScript to the Form’s show
event. A Form’s show
event executes after components are added to the page.Here’s what your final code should look like.
from ._anvil_designer import Form1Template
from anvil import *
from anvil.js.window import Quill
import anvil.js
class Form1(Form1Template):
def __init__(self, **properties):
self.init_components(**properties)
editor = anvil.js.get_dom_node(self.column_panel_1)
# add a ColumnPanel in the design view
# get the dom node for the ColumnPanel
self.quill = Quill( editor, {
'modules': { 'toolbar': True },
'theme': 'snow'
})
self.quill.on('text-change', self.text_change)
def text_change(self, delta, old_delta, source):
print(source, self.quill.getText())
The above code is enough to get started with a Quill editor in Anvil. Run the code!
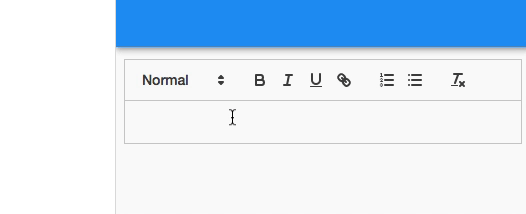
Copy The Example App
Click on the button below to clone a finished version of this app into your account.
Next up
Want more depth on this subject?
Read more about Accessing JavaScript.
Want another quickstart?
Every quickstart is on the Quickstarts page.
Do you still have questions?
Our Community Forum is full of helpful information and Anvil experts.