Hi,
I’m trying to have a Repeating Panel get filtered by a user’s Selected Value from a Drop Down.
In the screenshot below, the City field in the Repeating Panel is bound to the City field in a Data Table.
The City Drop Down however, is fed by a list of cities stored as a variable. I did originally have the City Drop Down bound to the City field in the Data Table, but I was getting repeating City values (i.e., San Francisco, San Francisco), when I only want unique values displayed.
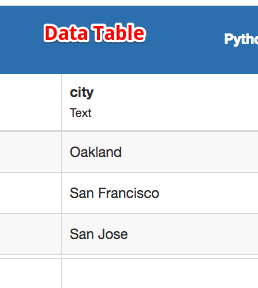
-
That said, any ideas on how to get the Repeating Panel to respond properly to the Drop Down?
-
And will I need to bind the Drop Down back to Data Table City field and solve separately for the repeating values issue?
Thank you! -Ian
I’m a bit short on time so this might not be the best quality answer you’ll get on this 
To get only unique values from a table into a drop down, you can do this (untested) :
results=app_tables.city_table.search()
cities=[]
for row in results:
if row['cityname'] not in cities:
cities.append(row['cityname'])
city_dropdown.items=cities
You can probably do that also in a comprehension.
You only need to set the items property for the repeating panel to update.
Argh - being called away, sorry - will come back to this later today if no one else has helped by then.
Hi David, thanks for replying even though you are short on time.
I don’t follow your solution 100% (I’m new to Python) but I do get that list comprehension can solve and that setting the item property on the repeating panel to update is necessary.
Below is the code I’ve written, but it’s throwing an error (‘Nonetype’ Object is not callable)
#all listings row code - this line works
self.repeating_panel_2.items=app_tables.job_listings.search()
#This code is not yet working
city_filtered_rows = [row for row in app_tables.job_listings.search()
if row[‘city’] == self.drop_down_2.selected_value()]
self.repeating_panel_2.items = city_filtered_rows
@ian.monat - That code is good! The only thing you’ve got wrong is that you’re calling self.drop_down_2.selected_value
as a function (it starts off with the value None
, hence the error when you try to call it). If you replaced that line with:
city_filtered_rows = [row for row in app_tables.job_listings.search()
if row[‘city’] == self.drop_down_2.selected_value]
self.repeating_panel_2.items = city_filtered_rows
…it should work!
(Sam has extended this code, so that if self.drop_down_2.selected_value
is None
, it shows you the whole table rather than showing you all the rows where city
is None
. You can choose which behaviour you want!)
Hi @meredydd, thanks for the snippets, they are having some effect but the issue is not solved, here’s what’s happening…
First, I decided to bind to drop_down_2 to the ‘city’ field in the data table instead of maintain a separate list of cities.
Second, I tried using Meredydd’s code because it was simpler, with the thought I could add Sam’s once it’s working. Here’s the code:
And here’s what it looks like when it runs:
The filter is working a little, only Oakland rows are displaying, but the first listing in the data table is in Oakland, so that is what the drop down is defaulting too, and if I select a different city from the drop down, I get this…
The repeating panel is no longer responding. Think a drop_down Change Event is required? Also, How can I get the drop down to default to all rows instead of those where city = Oakland? Thanks so much! -Ian
Sent via PM, thanks for taking a look!
Yes, code is working great, thanks so much!
I modified the code for the Job Category drop down, so now that’s working too, so both drop downs work correctly independently, but now when you try to use them both at once the results get weird (of course)
For example, if I select Engineering from job category, and that retrieves a job in San Francisco only,
then I select Oakland from the city drop down, the Oakland records override the Engineering records.
I think the drop_down_1 & drop_down_2 if statements are going to have to be more complex to handle this interaction? -Ian
Hey, I’m still working through this drop down issue, is what I’m trying to do here valid syntax?
I’m trying to set a variable to the results of an if statement and I don’t know if I’m just writing the if statement incorrectly, or if that’s simply not valid Python. Thanks! -Ian
Or you can use this form, equivalent to @Sam’s example:
variable = x if something else y
This is not always easier to read, but it’s good to know for use when you can’t use more lines of code, like in list comprehension or in the data binding formula.
Thanks, I got the filters to work! Woohoo! Ended up doing the inline statement.
Hi there -
my app is taking over 20 seconds to load, which makes it pretty unusable, and i placed some strategic print statements throughout my homepage and the clear culprit is these filters generating a list of values from the Anvil data tables, and there’s not a lot of rows in the tables, maybe only 20
Is there another way I can write this code that will take less time? Can I have the server do any work instead of the browser? These filters are more than half of the 20 second load time, so solving this will help a lot, here’s the code and what they look like:
#Job Category Drop Down
self.drop_down_category.items = [(‘All’,None)] + list(set([(row[‘job_category’]) for row in app_tables.job_listings.search()if row[‘active’] == True]))
self.drop_down_category_change()
#City Drop Down
self.drop_down_city.items = [(‘All’,None)] + list(set([(row[‘city’]) for row in app_tables.job_listings.search()if row[‘active’] == True]))
self.drop_down_city_change()
#Company Drop Down
self.drop_down_company.items = [(‘All’,None)] + list(set([(row[‘company’]) for row in app_tables.job_listings.search() if row[‘active’] == True]))
self.drop_down_company_change()
#Type drop down
self.drop_down_type.items = [(‘All’,None)] + list(set([(row[‘job_type’]) for row in app_tables.job_listings.search() if row[‘active’] == True]))
self.drop_down_company_change()
print(‘3’)
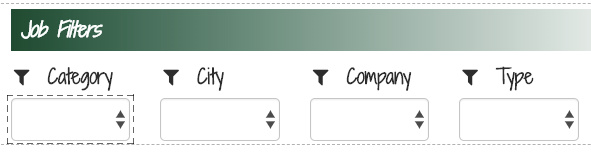
I would suggest something like the following (untested) to reduce the number of server round-trips when loading your app:
active_job_listings = [row for row in app_tables.job_listings.search() if row[‘active’]]
#Job Category Drop Down
self.drop_down_category.items = [(‘All’,None)] + [(row[‘job_category’]) for row in active_job_listings]
self.drop_down_category_change()
#City Drop Down
self.drop_down_city.items = [(‘All’,None)] + [(row[‘city’]) for row in active_job_listings]
self.drop_down_city_change()
#Company Drop Down
self.drop_down_company.items = [(‘All’,None)] + [row[‘company’] for row in active_job_listings]
self.drop_down_company_change()
#Type drop down
self.drop_down_type.items = [(‘All’,None)] + [row[‘job_type’] for row in active_job_listings]
self.drop_down_company_change()
Hope that helps!
Hi @daviesian, thanks for the tip, it did shave off a few seconds, but this block of code is still the bottleneck that is making the app load slowly, based on the print statements I’ve placed in the code
Any other ideas on how to speed it up…
-what about running any part of the code as a server call instead of in the browser?
-what about changing the placement of the code on the form (it’s currently toward the top, above the rest of the functions, except the __init__
of course
I’m all ears, thanks! -Ian
P.S. I did move some code to the server and have cut the page load time almost by half so far!